In this article we want to learn How to Create Responsive Navbar with CSS, responsive navbar is an essential part of any modern website. it provides easy navigation for users, regardless of the device they are using. in this article we want to talk how to create navbar.
How to Create Responsive Navbar with CSS
First let’s take look at the HTML structure for our navbar. we want to use an unordered list (ul) with list items (li) for each menu item. logo or site name will be placed in separate list item, followed by toggle button for small screens.
1 2 3 4 5 6 7 8 9 10 |
<nav> <ul class="navbar"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Courses</a></li> <li><a href="#">Video Courses</a></li> <li class="logo"><a href="#">Logo</a></li> <li class="toggle"><a href="#"><i class="fa fa-bars"></i></a></li> </ul> </nav> |
Now let’s add some CSS to style our navbar. we want to use use flexbox to create responsive layout. for small screens we will hide the menu items and show them when the toggle button is clicked.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
/* navbar styles */ .navbar { display: flex; justify-content: space-between; align-items: center; background-color: #333; color: #fff; padding: 10px; } .navbar li { list-style: none; margin: 0 10px; } .navbar li a { color: #fff; text-decoration: none; } .logo { font-size: 1.5em; } .toggle { display: none; } /* media query for small screens */ @media screen and (max-width: 768px) { .navbar { flex-wrap: wrap; } .navbar li { margin: 10px 0; width: 100%; } .toggle { display: block; order: 1; margin-left: auto; } .navbar .logo, .navbar li:not(.toggle) { display: none; } /* show menu items when toggle button is clicked */ .navbar li.toggle ~ li { display: block; } } |
In the CSS code we have set the display property of the navbar to flex and we have used justify-content and align-items to center the items horizontally and vertically. we also set the background color and font color. margin property is used to add space between the menu items.
For small screens we have used media query to set flex-wrap to wrap the items, and we set the margin to add space between the items. we hide the menu items except for the toggle button. when the toggle button is clicked, we use ~ selector to show the menu items.
This is the complete code and add this in html file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
<!DOCTYPE html> <html> <head> <title>Responsive Navbar</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <style> /* navbar styles */ .navbar { display: flex; justify-content: space-between; align-items: center; background-color: #333; color: #fff; padding: 10px; } .navbar li { list-style: none; margin: 0 10px; } .navbar li a { color: #fff; text-decoration: none; } .logo { font-size: 1.5em; } .toggle { display: none; } /* media query for small screens */ @media screen and (max-width: 768px) { .navbar { flex-wrap: wrap; } .navbar li { margin: 10px 0; width: 100%; } .toggle { display: block; order: 1; margin-left: auto; } .navbar .logo, .navbar li:not(.toggle) { display: none; } /* show menu items when toggle button is clicked */ .navbar li.toggle ~ li { display: block; } } </style> </head> <body> <nav> <ul class="navbar"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Courses</a></li> <li><a href="#">Video Courses</a></li> <li class="logo"><a href="#">Geekscoders</a></li> <li class="toggle"><a href="#"><i class="fa fa-bars"></i></a></li> </ul> </nav> </body> </html> |
This will be the result
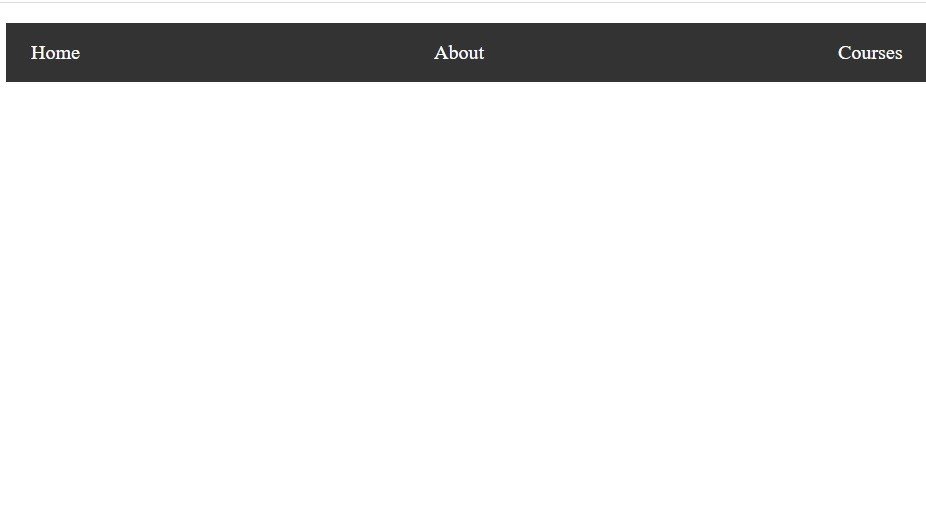
Learn More on CSS
- How to Center Text in CSS
- CSS Animation Tutorial
- CSS Grid Tutorial
- How to Use Flexbox in CSS
- How to Create CSS Dropdown Menu
- CSS Border Tutorial
- CSS Box Model Tutorial
- How to Style Form Elements with CSS
- How to Create CSS Tooltip
- CSS Typography Tutorial
- CSS Variable Tutorial
- CSS Hover Effect Tutorial
- How to Create CSS Slideshow