In this Flask CRUD Application lesson we are going to learn about Flask CRUD Application Body Design, basically in this lesson we want to design our body, we want to create a table for populating our data, but for right now it is just static data, till now we have not connected our Flask Application with MySQL database, also we are going to create some buttons for adding, deleting and updating employees.
Now open your index.html file and add this code, basically in this code we have a bootstrap table for populating the data with some buttons for adding, updating and deleting the data.
templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
{% extends 'base.html' %} {% include 'header.html' %} {% block title %}Flask CRUD Application {% endblock %} {% block body %} <div class="container"> <div class="row"> <div class="col md-12"> <div class="jumbotron p-3"> <h2>Manage <b>Employees </b> <button type="button" class="btn btn-success float-right" data-toggle="modal" data-target="#mymodal">Add New Employees</button> </h2> <table class="table table-hover table-dark"> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Phone</th> <th>Action</th> </tr> <tr> <td>01</td> <td>Geekscoders</td> <td>geeks@gmail.com</td> <td>858558</td> <td> <a href="" class="btn btn-warning btn-xs" data-toggle="modal" data-target="#modaledit"> Edit</a> <a href="" class="btn btn-danger btn-xs" onclick="return confirm('Are You Sure To Delete ?')"> Delete </a> </td> </tr> </table> </div> </div> </div> </div> {% endblock %} |
This is our App.py file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
from flask import Flask, render_template #create the object of Flask app = Flask(__name__) #creating our routes @app.route('/') def Index(): return render_template("index.html") #run flask app if __name__ == "__main__": app.run(debug=True) |
templates/base.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css"> <meta charset="UTF-8"> <title>{% block title %} {% endblock %} </title> </head> <body> {% block body %} {% endblock %} <script src="https://code.jquery.com/jquery-3.4.1.slim.min.js"> </script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"> </script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js"> </script> </body> </html> |
templates/header.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
{% extends 'base.html' %} {% block title %} Flask CRUD {% endblock %} {% block body %} <div class="jumbotron p-3"> <div class="well text-center"> <h1>Flask CRUD Application - Geekscoders.com</h1> </div> </div> {% endblock %} |
Run your application and go to http://localhost:5000/, this will be the result.
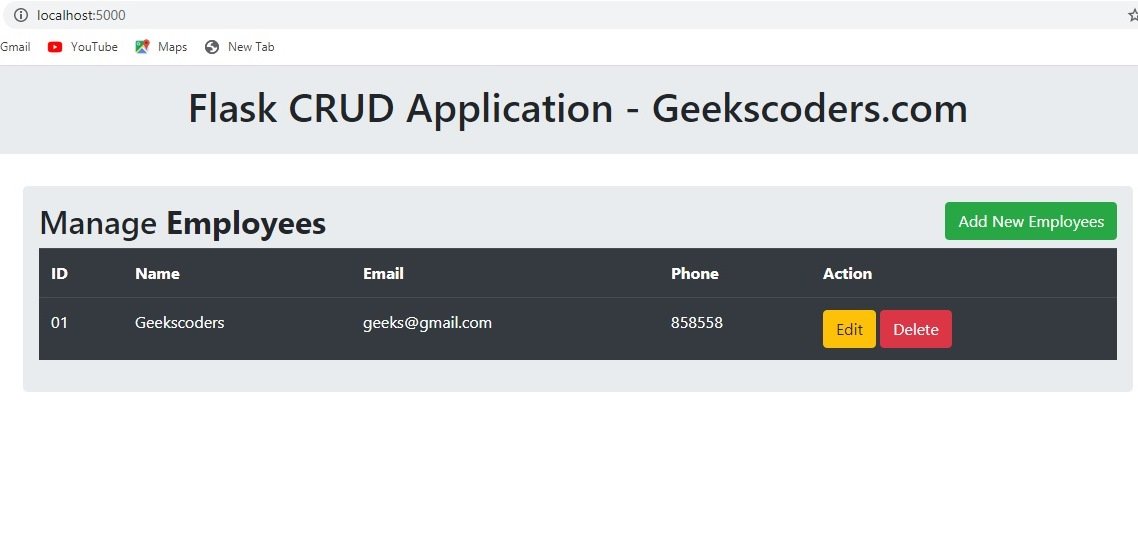