In this Flask CRUD Application lesson we are going to learn about Flask CRUD Application Creating MySQL Database, for creating of the MySQL database we are going to use WAMP Server, so make sure that you have already installed WAMP Server.
Open your WAMP Server and iam going to call my database CRUD, for right now iam not going to create tables for my newly created database, i will add the tables dynamically using SQLAlchemy, as we have already said that in this course we want to use SQLAlchemy.
You need these installations.
1 |
ip install Flask-SQLAlchemy |
1 |
pip install mysqlclient |
Now open your App.py file and you need to add some codes for database configuration and database tables.
These configurations are for database connection, you need to give the database type, database name, password and host name.
1 2 3 4 5 |
app.config['SQLALCHEMY_DATABASE_URI'] = 'mysql://root:''@localhost/crud' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False db = SQLAlchemy(app) |
This is our database table.
1 2 3 4 5 6 7 8 9 10 |
class Data(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100)) email = db.Column(db.String(100)) phone = db.Column(db.String(100)) def __init__(self, name, email, phone): self.name = name self.email = email self.phone = phone |
And this is our complete code for App.py file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
from flask import Flask, render_template from flask_sqlalchemy import SQLAlchemy #create the object of Flask app = Flask(__name__) app.secret_key = "geekscoderssecretkey" #SqlAlchemy Database Configuration With Mysql app.config['SQLALCHEMY_DATABASE_URI'] = 'mysql://root:''@localhost/crud' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False db = SQLAlchemy(app) # Creating model table for our CRUD database class Data(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100)) email = db.Column(db.String(100)) phone = db.Column(db.String(100)) def __init__(self, name, email, phone): self.name = name self.email = email self.phone = phone #creating our routes @app.route('/') def Index(): return render_template("index.html") #run flask app if __name__ == "__main__": app.run(debug=True) |
After that you need to open your Python terminal in Pycharm IDE.
1 2 |
>>> from App import db >>> db.create_all() |
Now our database table is created.
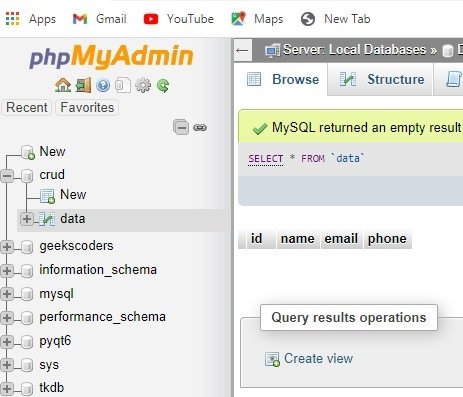
This is our other files.
templates/base.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css"> <meta charset="UTF-8"> <title>{% block title %} {% endblock %} </title> </head> <body> {% block body %} {% endblock %} <script src="https://code.jquery.com/jquery-3.4.1.slim.min.js"> </script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"> </script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/js/bootstrap.min.js"> </script> </body> </html> |
templates/header.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
{% extends 'base.html' %} {% block title %} Flask CRUD {% endblock %} {% block body %} <div class="jumbotron p-3"> <div class="well text-center"> <h1>Flask CRUD Application - Geekscoders.com</h1> </div> </div> {% endblock %} |
templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 |
{% extends 'base.html' %} {% include 'header.html' %} {% block title %}Flask CRUD Application {% endblock %} {% block body %} <div class="container"> <div class="row"> <div class="col md-12"> <div class="jumbotron p-3"> <h2>Manage <b>Employees </b> <button class="btn btn-success float-right" data-target="#mymodal" data-toggle="modal" type="button">Add New Employees </button> </h2> <table class="table table-hover table-dark"> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Phone</th> <th>Action</th> </tr> <tr> <td>01</td> <td>Geekscoders</td> <td>geeks@gmail.com</td> <td>858558</td> <td> <a class="btn btn-warning btn-xs" data-target="#modaledit" data-toggle="modal" href=""> Edit</a> <a class="btn btn-danger btn-xs" href="" onclick="return confirm('Are You Sure To Delete ?')"> Delete </a> </td> </tr> <!-- Modal Edit Employee--> <div class="modal fade" id="modaledit" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h4 class="modal-title">Update Information</h4> </div> <div class="modal-body"> <form action="" method="POST"> <div class="form-group"> <label>Name:</label> <input name="id" type="hidden" value=""> <input class="form-control" name="name" type="text" value="Geekscoders"> </div> <div class="form-group"> <label>Email:</label> <input class="form-control" name="email" type="text" value="geeks@gmail.com"> </div> <div class="form-group"> <label>Phone:</label> <input class="form-control" name="phone" type="text" value="858558"> </div> <div class="form-group"> <button class="btn btn-primary" type="submit">Update</button> </div> </form> </div> <div class="modal-footer"> <button class="btn btn-secondary" data-dismiss="modal" type="button">Close</button> </div> </div> </div> </div> </table> </div> <!-- Modal Add Employee--> <div class="modal fade" id="mymodal" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h4 class="modal-title">Add Employee</h4> </div> <div class="modal-body"> <form action="" method="POST"> <div class="form-group"> <label>Name:</label> <input class="form-control" name="name" required="1" type="text"> </div> <div class="form-group"> <label>Email:</label> <input class="form-control" name="email" required="1" type="email"> </div> <div class="form-group"> <label>Phone:</label> <input class="form-control" name="phone" required="1" type="number"> </div> <div class="form-group"> <button class="btn btn-primary" type="submit">Add Employee</button> </div> </form> </div> <div class="modal-footer"> <button class="btn btn-secondary" data-dismiss="modal" type="button">Close</button> </div> </div> </div> </div> </div> </div> </div> {% endblock %} |