In this Python Selenium lesson we want to learn about Selenium Mouse Based Action Chains in Python. There are around eight different mouse actions that can be performed using the Action Chains class, we have already saw some of them, like clicking on the elements, in here we want to learn about some more Mouse Based Action Chains in Python Selenium.
move_by_offset()
The move_by_offset() method is used to move the mouse from its current position to another point on the web page. Developers can specify the X distance and Y distance the mouse has to be moved.
So first of all we need a web page to do our automation, we are using a code from JqueryUI and it is selectable code, you can get the code from their or you can just create an html file and add this code, you can see that we have added name attributes for our five items in the list, because we want to select these five items, and we want to use the find_element_by_name() locator from selenium.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>jQuery UI Selectable - Display as grid</title> <link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <link rel="stylesheet" href="/resources/demos/style.css"> <style> #feedback { font-size: 1.4em; } #selectable .ui-selecting { background: #FECA40; } #selectable .ui-selected { background: #F39814; color: white; } #selectable { list-style-type: none; margin: 0; padding: 0; width: 450px; } #selectable li { margin: 3px; padding: 1px; float: left; width: 100px; height: 80px; font-size: 4em; text-align: center; } </style> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <script> $( function() { $( "#selectable" ).selectable(); } ); </script> </head> <body> <ol id="selectable"> <li class="ui-state-default" name="one">1</li> <li class="ui-state-default" name = "two">2</li> <li class="ui-state-default" name = "three">3</li> <li class="ui-state-default" name = "four">4</li> <li class="ui-state-default" name="five">5</li> <li class="ui-state-default">6</li> <li class="ui-state-default">7</li> <li class="ui-state-default">8</li> <li class="ui-state-default">9</li> <li class="ui-state-default">10</li> <li class="ui-state-default">11</li> <li class="ui-state-default">12</li> </ol> </body> </html> |
And this is the Python code, you can see that first of all we have located the web element in page, in this example i want to move my mouse from the third web element by 200 in the X and 200 in Y directions. and you can see that we have used move_by_offset() and you need to give the X position and Y position.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains driver = webdriver.Chrome() driver.get('C:/selectable.html') three = driver.find_element_by_name('three') l = three.location s = three.size actions = ActionChains(driver) actions.move_by_offset(200,200) actions.click().perform() print(f"Cordinates : {l}" ) print(f"Size : {s}") |
Click
We have already saw clicking and it is used for clicking on the web elements in a web page. in this example first we have located our three web elements by name attribute, after that we have created our ActionChains object and at the end we have used the click method for clicking of the three web elements in the web page.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains driver = webdriver.Chrome() driver.get('C:/selectable.html') one = driver.find_element_by_name('one') two = driver.find_element_by_name('two') three = driver.find_element_by_name('three') actions = ActionChains(driver) actions.click(one) actions.click(two) actions.click(three) actions.perform() |
Run the code and it will select the web elements from one up to three.
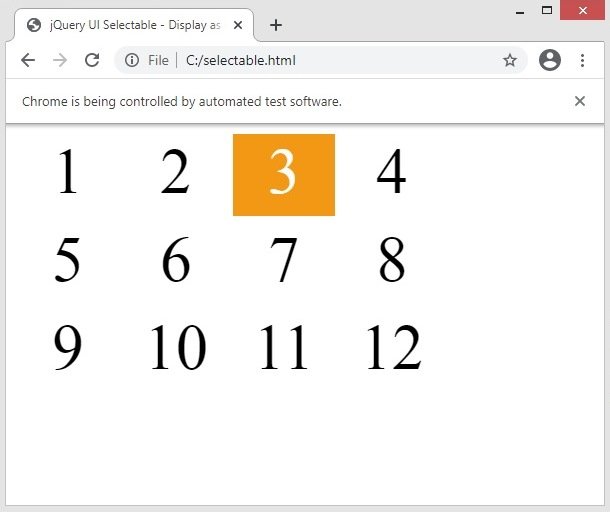
click_and_hold()
The click_and_hold() method is another method of the Actions class that left-clicks on an element and holds it without releasing the left button of the mouse. This method will be useful when executing operations such as drag-and-drop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains driver = webdriver.Chrome() driver.get('C:/sortable.html') one = driver.find_element_by_name("one") four = driver.find_element_by_name("four") actions = ActionChains(driver) actions.click_and_hold(one) actions.release(four) actions.perform() |
In the above example we want to hold the first web element and release that in the place of fourth web element, you can see that we have used click_and_hold() method, also we have used release() method for the releasing of the web elements.
Run the code and this is the result.
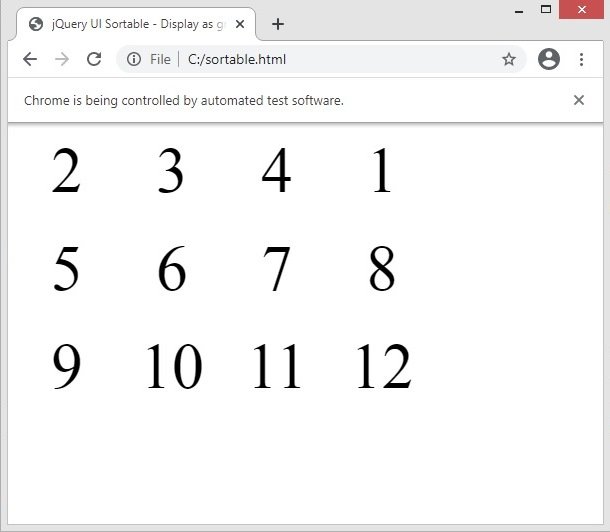
Drag and Drop in Python Selenium
Now we are going to create Drag and Drop in Python Selenium, there are two ways that you can do this, the first one is that you can use click_and_hold() method and the second way is the drag_and_drop() method.
So first let’s used click_and_hold() method for creating of drag and drop in Python Selenium. in this code first we have located our web elements using id, after that we have used our click_and_hold() method with release().
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains driver = webdriver.Chrome() driver.get('https://demoqa.com/droppable') dragged = driver.find_element_by_id("draggable") dropped = driver.find_element_by_id("droppable") actions = ActionChains(driver) actions.click_and_hold(dragged) actions.release(dropped) actions.perform() |
There might be many instances where we may have to drag-and-drop components or WebElements of a web page. now we have saw that how you can use click_and_hold() for drag and drop, but there is a method of drag_and_drop() in selenium that you can use.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains driver = webdriver.Chrome() driver.get('https://demoqa.com/droppable') src = driver.find_element_by_id("draggable") trgt = driver.find_element_by_id("droppable") actions = ActionChains(driver) actions.drag_and_drop(src, trgt) actions.perform() |
In the above example we have used drag_and_drop() method, you need to give the source and target for drag and drop.
Run the code ad this is the result.
