In this lesson i want to show you how to Create First GUI Application using Python Tkinter,
Tkinter is a standard Python library that is used to create graphical user interfaces (GUIs) for desktop applications. Tkinter provides a set of tools for creating a wide variety of graphical elements, such as windows, buttons, labels, and text boxes, among others. It also provides a way to handle and respond to user input, such as button clicks and key presses.
Tkinter is built on top of the Tcl/Tk GUI toolkit and provides a Pythonic interface to it. This means that it provides a set of Python classes and methods that correspond to the different widgets and functionality provided by Tcl/Tk. This allows Python developers to create GUI applications using the same familiar and easy-to-use syntax that they use for other parts of their code.
Tkinter is a simple and easy to use toolkit and is a good choice for small to medium-sized projects or for beginners who are just learning how to create GUI applications. However, for more advanced or large projects, other toolkits such as PyQt or wxPython may be more suitable.
Overall Tkinter is a widely used library for building GUI application in python, it’s simple, easy to use and ships with python.
Learn More on TKinter
Here is an example of a simple GUI application using the Tkinter library in Python:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import tkinter as tk # Create the main window root = tk.Tk() root.title("My First GUI App") # Add a label to the window label = tk.Label(root, text="Hello World!") label.pack() # Add a button to the window button = tk.Button(root, text="Click Me!", command=quit) button.pack() # Run the main loop root.mainloop() |
This code creates a Tkinter window with a title “My First GUI App”, a label that says “Hello World!”, and a button that says “Click Me!”. The button has a command that will close the window when clicked.
Here’s a brief explanation of the code:
import tkinter as tk
: imports the Tkinter library and gives it the alias “tk”root = tk.Tk()
: creates the main window of the applicationroot.title("My First GUI App")
: sets the title of the windowlabel = tk.Label(root, text="Hello World!")
: creates a label widget with the text “Hello World!” and places it in the main windowlabel.pack()
: packs the label widget into the main window, which makes it visiblebutton = tk.Button(root, text="Click Me!", command=quit)
: creates a button widget with the text “Click Me!” and assigns the functionquit
as the command to be executed when the button is clicked.button.pack()
: packs the button widget into the main window, which makes it visibleroot.mainloop()
: runs the Tkinter event loop, which is responsible for handling user input and updating the GUI.
This is just a basic example, you can add more widgets and more functionalities to the application. Tkinter has a wide variety of widgets such as buttons, labels, text boxes, checkboxes, and more that you can use to create more complex applications.
Hope this example helps you to get started with Tkinter. Let me know if you have any other questions.
Run the complete code and this will be the result.
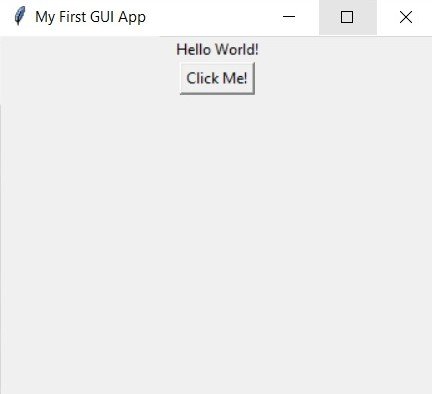