In this Python OpenCV lesson we are going to learn about Python OpenCV Circle Detection, so OpenCV has a function for detecting circles, called HoughCircles. It works in a very similar fashion to HoughLines, but where minLineLength and maxLineGap were the parameters to discard or retain lines, HoughCircles has a minimum distance between circles’ centers, minimum, and maximum radius of the circles. Here’s the obligatory.
This is the complete code for this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import cv2 import numpy as np planets = cv2.imread("planet.jpg") gray = cv2.cvtColor(planets, cv2.COLOR_BGR2GRAY) img =cv2.medianBlur(gray, 5) cimg = cv2.cvtColor(img, cv2.COLOR_GRAY2BGR) circles = cv2.HoughCircles(img, cv2.HOUGH_GRADIENT, 1,120, param1=100, param2=30, minRadius=0, maxRadius=0) circles = np.uint16(np.around(circles)) for i in circles[0, :]: # Outer Circle cv2.circle(planets, (i[0], i[1]),i[2], (0,255,0), 2) # Center of the circle cv2.circle(planets, (i[0], i[1]), 2, (0, 0, 255), 5) cv2.imshow("Circle Detection", planets) cv2.waitKey(0) cv2.destroyAllWindows() |
We want to use this image for circle detection.
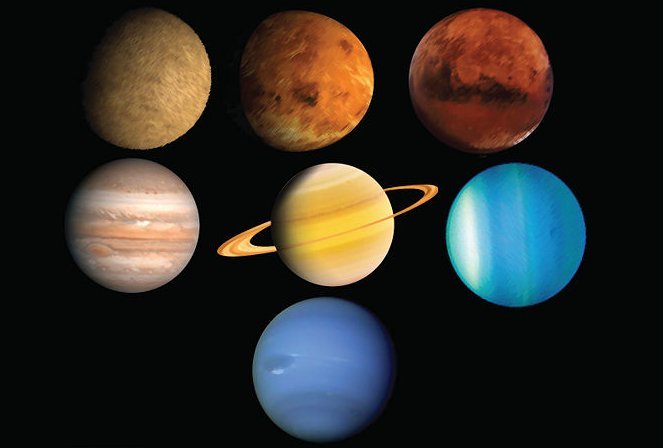
So this is our HoughCircle algorithm for circle detection, it needs some parameters like input image, dp, minDistance, minRadious and maxRadious.
1 2 3 |
circles = cv2.HoughCircles(img, cv2.HOUGH_GRADIENT, 1,120, param1=100, param2=30, minRadius=0, maxRadius=0) circles = np.uint16(np.around(circles)) |
This line of code is for drawing two circles, an outer big circle and a inner small circle.
1 2 3 4 5 6 7 8 |
for i in circles[0, :]: # Outer Circle cv2.circle(planets, (i[0], i[1]),i[2], (0,255,0), 2) # Center of the circle cv2.circle(planets, (i[0], i[1]), 2, (0, 0, 255), 5) |
Run the complete code and this is the result.
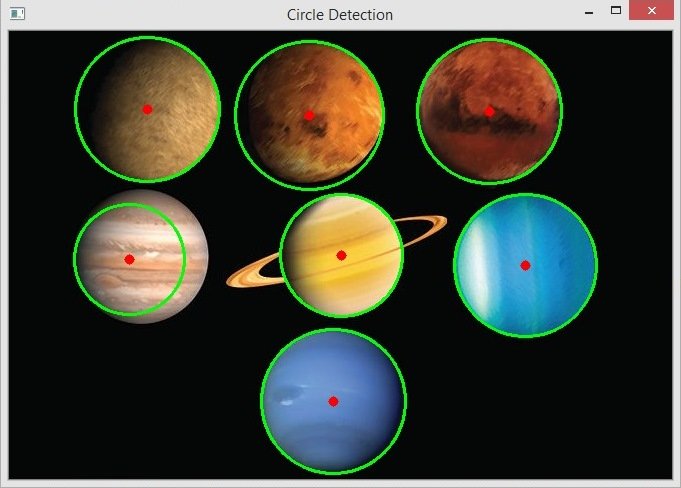