In this wxPython article we want to learn about How to Create wxPython Static Text with wx.StaticText, so wxPython is popular GUI library for Python that allows developers to create cross platform applications with native look and feel. one of the most basic components of any GUI application is static text, which is used to display text that does not change. in this article we want to talk how to create wxPython static text with wx.StaticText.
First of all we need to install wxPython and you can use pip for that
1 |
pip install wxpython |
wx.StaticText Basics
wx.StaticText is a class that is used to create static text in wxPython. constructor for wx.StaticText takes two arguments, parent window and label. parent window is the window in which the static text will be displayed. label is the text that will be displayed.
1 2 3 4 5 6 7 8 9 10 11 |
import wx app = wx.App() frame = wx.Frame(None, title='Static Text Example') panel = wx.Panel(frame) static_text = wx.StaticText(panel, label='Welcome to geekscoders.com') frame.Show() app.MainLoop() |
In the above code we have created wxPython application with frame and panel. after that we creates wx.StaticText object with panel as the parent window and also label. we then show the frame and start wxPython event loop.
Run the complete code and this will be the result
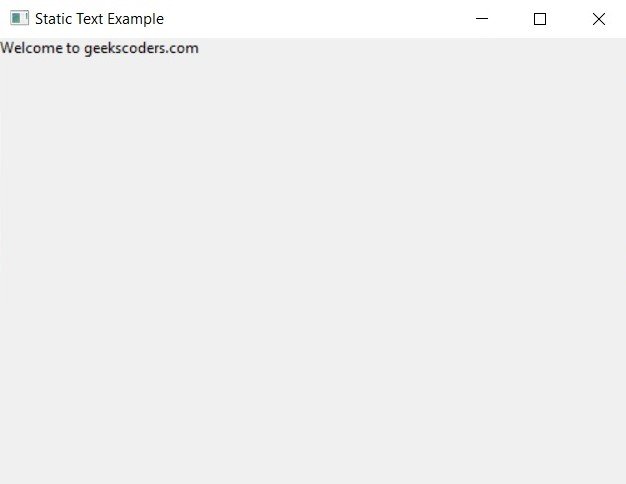
Now let’s do our styling, you can style wx.StaticText objects with different properties such as font, color, alignment and border. this is an example of how to set the font, color and alignment of wx.StaticText:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import wx app = wx.App() frame = wx.Frame(None, title='Static Text Example') panel = wx.Panel(frame) static_text = wx.StaticText(panel, label='Welcome to geekscoders.com') static_text.SetFont(wx.Font(18, wx.FONTFAMILY_DEFAULT, wx.FONTSTYLE_NORMAL, wx.FONTWEIGHT_BOLD)) static_text.SetForegroundColour(wx.Colour(255, 0, 0)) static_text.SetBackgroundColour(wx.Colour(255, 255, 255)) static_text.SetWindowStyle(wx.ALIGN_CENTER_HORIZONTAL|wx.ALIGN_CENTER_VERTICAL) frame.Show() app.MainLoop() |
In the above code we have created wx.StaticText object with larger font, red foreground color, white background color and centered alignment. we have used SetFont() method to set the font, SetForegroundColour() method to set foreground color, SetBackgroundColour() method to set the background color and SetWindowStyle() method to set the alignment.
Run the complete code and this will be the result
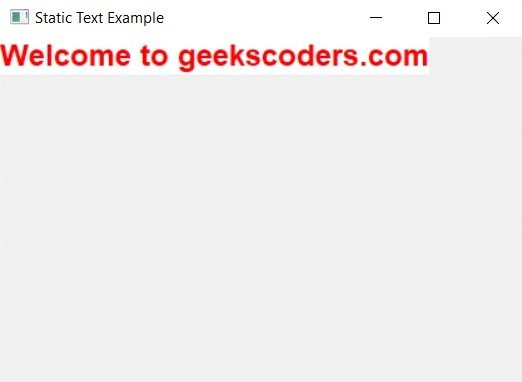