In this Python programming article we are going to talk about Introduction to Object-Oriented Programming in Python, we will cover different topics on Python Object Oriented Programming, from creating classes and objects, up to class inheritance in Python.
Introduction to Object-Oriented Programming in Python
As you know Python is object oriented programming language, it means that we can solve a problem in Python language by creating classes and objects in our program. so we can say that object oriented programming is a computer programming model that arranges software design with data, or objects, rather than functions. there are different concepts that you should know in object oriented programming like creating classes, creating object, and there are some object oriented principles like inheritance, polymorphism, abstraction and encapsulation, particularly in this video we want to learn how you can create classes and how you can instantiate a class in Python.
What are Python Classes in OOP ?
In Python Object Oriented Programming [ Python OOP ] a class is a blueprint for the objects. or we can say that a class is a way of organizing information about a type of data, by creating a class we can reuse elements when making multiple instances of that data type for example, we create a class Car, now if we want to create the instances of this car class, it may be a BMW car or Ferrari car or another type of car. the Car class would allow you to store similar information that is unique to each car (they are different models, and maybe different colors, etc.) and associate the appropriate information with each car.
So now let’s create simple Python classes example, you can use class keyword followed by the name of the class in Python. in the example we have created a simple class at name of MyClass, right now we are not adding anything the class body.
1 2 |
class MyClass: pass |
- TKinter Tutorial For Beginners
- PyQt5 Tutorial – Build GUI in Python with PyQt5
- Python Speech Recognition For Beginners
If you want to use this Python OOP class you need to instantiate the objects from this class, and that is called Python objects, creating of instance class is simple, you can just give the name of the class followed by pair of parenthesis.
1 2 3 4 5 6 |
class MyClass: pass a = MyClass() b = MyClass() |
Add Attributes in Python Classes
Right now we are not going to do anything in the class body itself, we want to add some attributes to a created object of the class. we have created a simple class, and after that we have instantiated two objects from the class, after that we have assigned some attributes to the class using dot notation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class Student: pass st1 = Student() st2= Student() st1.name = "Parwiz" st1.age = 25 st2.name = "Geekscoders" st2.age = 20 print(st1.name, st1.age) print(st2.name, st2.age) |
Run the code and you will see this result.

Python Initializer Method
Most object oriented programming languages have the concept of a constructor, special method that creates and initializes the object when it is created, now in python we have initializer method this is a special method and it is called when an object is created from a class and it allows the class to initialize the attributes of the class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
class Student: def __init__(self, name, email): self.name = name self.email = email st1 = Student("Geekscoders", 'geekscoders@gmail.com') st2 = Student('Parwiz', 'par@gmail.com') print(st1.name) print(st1.email) print(st2.name) print(st2.email) |
As you can see in the above example we have created an initializer method, and the self represents the instance of the class. By using the “self” keyword we can access the attributes and methods of the class in python, when you want to create the instance of the class you need to pass the name and email in the class.
Python Class Attributes
Class attribute is a Python variable that is owned by a class rather than a particular object. It is shared between all the objects of this class and it is defined outside the initializer method of the class.
Python Instance Attributes
An instance attribute is a Python variable belonging to only one object. This variable is only accessible in the scope of this object and it is defined inside the init() method also Instance Attributes are unique to each object.
Now let’s take a look at this example, in here the email is a class attribute and it belongs to the class, it is shared by all instances. When you change the value of a class attribute, it will affect all instances that share the same exact value. the variable name and age are instance variable, because they are unique to the instance, if you change one instance variable, the second instance variable will not be affected. but in class attribute when you change the variable, it will affect all the instances.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class Student: email = 'geekscoders@gmail.com' def __init__(self, name, age): self.name = name self.age = age st1 = Student("Geekscoders", 20) st2 = Student('Parwiz', 25) print(st1.name) print(st1.age) print(st1.email) print(st2.name) print(st2.age) print(st2.email) |
Add Python Methods in Python Classes
Now let’s add method in the class, take a look at this example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class Student: def __init__(self, name, age, email): self.name = name self.age = age self.email = email def print_info(self): print(f"My name is {self.name} My Age is " f"{self.age} and My email is {self.email} ") st1 = Student("GeeksCoders", 20, "geekscoders@gmail.com") st1.print_info() |
Python Class Inheritance
Now we are going to talk about Python Class Inheritance, Class inheritance is one of the most important concepts in Object Oriented Programming, if you are familiar with other OOP programming languages like Java or C++, so it has the same concepts, basically a class inheritance allows us to create a relationship between two or more classes. now in object oriented programming one class can inherit attributes and methods from another class.
So now let’s take a look at this image example.

If you take a look at the above image, we have created two classes, the first class is Class A, in this class we have created two variables and also we have a method. in the term of Object Oriented Programming, we can call this class as base class, parent class or super class. we have another class that is Class B, and this class inherited from the Class A, for this class we can call derived class, child class or sub class. now in class inheritance when a class extends from another class, the derived class can access to all attributes and methods of the base class. for example in here our Class B can access to the fname, lname and full_name() method of our Class A or base class.
Let’s create a practical example in Python Class Inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
class Person: def __init__(self, name, email): self.name = name self.email = email def full_info(self): print(f'Name Is {self.name} And Email Is {self.email}') class Student(Person): pass stu1 = Student("John", 'john@gmail.com') stu2 = Student("Geekscoders", 'coders@gmail.com') stu1.full_info() stu2.full_info() |
In the above code we have a base class and we have a derived class, in the base class we have some instance attributes and a method for printing the variables. now our derived class can access to all attributes and methods of the base class, for example Student class can access to the name, email variables and also full_info() method.
You can see that in here we have created the instance for our Student Python class not Person class, and in the Student class we don’t have any attributes like name and email or a method for printing the information, these are added in the Person class.
1 2 3 4 |
stu1 = Student("John", 'john@gmail.com') stu2 = Student("Geekscoders", 'coders@gmail.com') stu1.full_info() stu2.full_info() |
Run the code and this will be the result.

Python Super() Function
Now we want to talk aboutSuper() Function in Python, Python Super() Function is used for accessing to the methods and properties of the parent class or base class, and super function returns a proxy object.
Now let’s create a practical example on Python Super() function.
1 2 3 4 5 6 7 8 9 10 11 |
class Person: def __init__(self): print("Geekscoders - Base Class Person") class Student(Person): def __init__(self): print("Geekscoders - Derived Class Student ") st = Student() |
in the above code we have two classes, the first class is Person class and it is our parent class, we have our initializer method in this class, also in the initializer we are just printing some texts in the console. the second class is Student class and it is a child class of the Person class, and in here also we are going to print something in the console. now if you run this code you will see that it is not calling the base class initializer method, but it is calling the derived class initializer method.
This is the result and as we can see it is calling the derived class initializer method.

Now what if we want to call our base class initializer method, for that we are going to use the super function in Python programming, let’s bring changes to our code and add the super function.
1 2 3 4 5 6 7 8 9 10 11 12 |
class Person: def __init__(self): print("Geekscoders - Base Class Person") class Student(Person): def __init__(self): super().__init__() print("Geekscoders - Child Class Student") st = Student() |
Run the code and you will see that it is calling the base class initializer method.

Let’s create another example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
class Person: def __init__(self, name, age): self.name = name self.age = age class Student(Person): def __init__(self, name, age, email): super().__init__(name, age) self.email = email st = Student("Geekscoders", 20, "geekscoders@gmail.com") print(st.name) print(st.age) print(st.email) |
In this code, Person is a super (parent) class, while Student is a derived (child) class. The usage of the super function allows the child class to access the parent class’s init() property. In other words, super() allows you to build classes that easily extend the functionality of previously built classes without implementing their functionality again.
Run the code and this is the result.
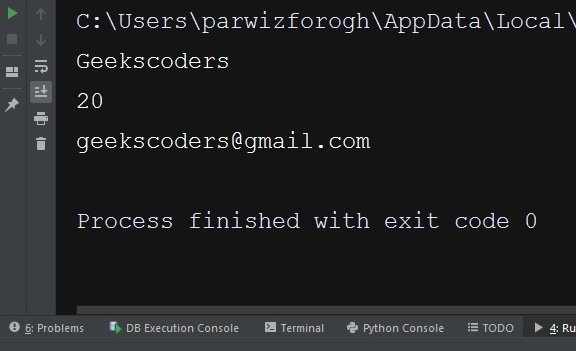
Python Method Overriding
Now we want to talk about Method Overriding in Python, so method overriding is used for changing the implementation of a method provided by one of it is parent or base class.
OK let’s create our practical example on Method Overriding in Python classes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class Person: def full_name(self): print("My name is Geekscoders") class Student(Person): def full_name(self): print("My name is Parwiz") p = Person() p.full_name() st = Student() st.full_name() |
So in the above example we have two classes, the first class is our Person Class and it is our base class, also we have added a method of def full_name() , on the other hand we have another class at name of Student class and this class is extending from the base class, so for this we can say that it is a derived class, now you can see that we have the same name method in our derived class, when you have two methods with the same name and different implementation that is called Method Overriding, and these two methods have their own implementation, even tough they have the same name but the implementations are different.
If you run the code you can see that we have different implementation for the same method name.
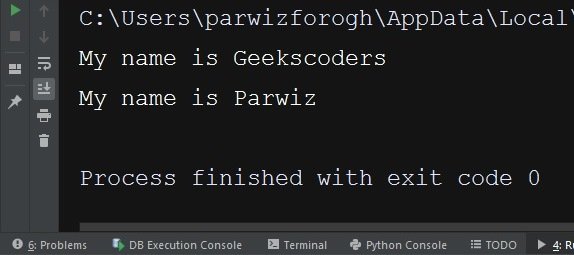
Python Multiple Inheritance
In this second we want to talk aboutMultiple Inheritance in Python, so first of all what is Multiple Inheritance in Python, if a class extends from more than one class, that is called multiple inheritance.
Now let’s look at this Inheritance image example.

In the above image we have three classes, Class A and Class B are our base class, also we have another class that is Class C, now this Class C extends from Class A and Class B, as we have already said that if a class extends from more than one class, that is called multiple inheritance. in here our C Class extends from two base classes, now the C class can access to all attributes and methods of the Class A and Class B.
Let’s create a practical example for Multiple Inheritance in Python programming language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class A: def print_a(self): print("Iam Class A Method") class B: def print_b(self): print("Iam Class B Method ") class C(A,B): pass c = C() c.print_a() c.print_b() |
In the above example we have two base classes and the third class is extending from these two base class, and if you see in our base classes we have methods, after extending Class C can access to these two methods.
Run the code and this is the result.

Let’s create another example, in this example we want to override a method in to our base classes, also we are going to talk about Method Resolution Order (MRO).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class A: def full_name(self): print("This is my full name - Class A") class B: def full_name(self): print("This is my full name - Class B") class C(B, A): pass c = C() c.full_name() |
You can see that when we are extending our Class C, we have the order of B and A, now it will first check the method in the C class, if it does not find the method in the C class, than it checks the B Class, now it means that the order matters in Python Multiple Inheritance, if we run this code you will see that first we have our B Class method.
Python Multi Level Inheritance
In this section we want to learn about Multi Level Inheritance in Python, so in object oriented programming when you inherits a derived class from another derived class this is called multi level inheritance and it can be done at any depth.
Now let’s take a look at this Inheritance image in Python OOP.

If you see in the above image we have three classes, we have a Class A, this class is our base class. we have another Class B, this class extends from the base Class A. we have another Class C, now this class extends from Class B. as we have already said when you extends a derived class from another derived class this is called multi level inheritance and it can be done at any depth. now our Class B can access to all attributes and method of the Class A, and our Class C can access to all attributes and methods of the Class A and Class B.
Now let’s create a practical example, in this example we have our base class that is class Person, in this class we have created a method. we have two more Python classes, one is class Student and it is extending from class Person, there is a Python method in this class. also we have another class that is class Programmer, this class extends from the Student class. now if you create the instance of the Programmer class, this class can access to the method of the Person and Student classes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
class Person: def per_method(self): print("Iam a Person") class Student(Person): def stu_method(self): print("Iam a Student") class Programmer(Student): pass pro = Programmer() pro.per_method() pro.stu_method() |
Now run your code and this will be the result.

As i have already said you can create Python Multi Level Inheritance at any depth.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
class Person: def per_method(self): print("Iam a Person") class Student(Person): def stu_method(self): print("Iam a Student") class Programmer(Student): def pro_method(self): print("Iam a Programmer") pass class D(Programmer): pass pro = Programmer() pro.per_method() pro.stu_method() d = D() d.per_method() d.stu_method() d.pro_method() |
Python Hierarchical Inheritance
In this part we want to learn about Hierarchical Inheritance in Python, so in python object oriented programming When more than one derived classes are created from a single base class that is called hierarchical inheritance.
Now let’s take a look at this Inheritance image in Python classes.

If you see in the image, we have four classes, Class A is our base or parent class, we have another three classes and all of them are extending from just one base class, as i have already said When more than one derived classes are created from a single base class that is called hierarchical inheritance. now there is a relationship between Class A and three derived classes(B, C, D), but there is no relationship between the derived classes, it means that we have a relationship between base class and derived classes, but there is no relationship between derived classes, for example we don’t have any relationship between Class B, Class C and Class D.
Now let’s create practical example in Python Hierarchical Inheritance
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
class Animal: def run(self, name): self.name = name print(f'{self.name} Is Runing') class Dog(Animal): pass class Cat(Animal): pass dog = Dog() dog.run("Dog") cat = Cat() cat.run("Cat") |
In the example we have three python classes, class Animal is our base class and we have two more classes that are inheriting from just one base class. we have relationship between Class Animal and Class Cat, also between Class Animal and Class Dog, but there is no relationship between Class Cat and Class Dog.
Run the code and this is the result.
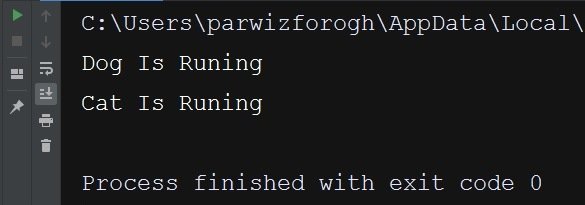
So we can say that understanding object-oriented programming (OOP) in Python is essential for creating efficient and scalable code. by using OOP concepts like encapsulation, inheritance, abstraction and polymorphism, developers can write code that is more modular, reusable and easier to maintain.
In this tutorial we have learned the basics of OOP in Python including creating classes, objects and methods. we also looked at how to use inheritance and polymorphism to extend and modify existing classes. if you are beginner or an experienced Python programmer, understanding OOP is critical skill to have in your toolkit. by mastering these concepts, you will be able to write code that is more organized, efficient and easier to maintain.
So if you are looking to take your Python programming skills to the next level, be sure to dive deeper into OOP and explore how you can incorporate these concepts into your own projects. by leveraging the power of Python object-oriented features, you will be able to write more robust and scalable code that can help you achieve your goals.