In this Python File Handling tutorial we want to learn Reading File in Python, if you want to work on the files, the first thing is that you need to open that file, even if you want to read the content of the file, than you need to open the file, you can use open() function for opening the file.
Now let’s create an example, first you need to create a txt file and add some texts, as i have already created a txt file and added some texts in that file.
1 2 |
Hello Friends, How Are You Welcome to Geekscoders website |
Create a new Python file and add this code. in this code first of all we need to open our text file, make sure that you have already created a file in your working directory, we have used open() method for opening the file in Python, the open() function returns an object representing the file. after that you can use read() method for reading the contents of the text file.
1 2 3 |
with open('mfile.txt') as file_object: contents = file_object.read() print(contents) |
Run the code and this is the result.

Right now our file is in our directory, if the text file is located in another place, for example in your drives you can just give the path of the file.
1 2 3 |
with open('C:/mfile.txt') as file_object: contents = file_object.read() print(contents) |
Also you can read a text file contents line by line.
1 2 3 4 5 |
file_name = 'mfile.txt' with open(file_name) as file_object: for line in file_object: print(line) |
Run the code and you can see that we have more blank spaces.
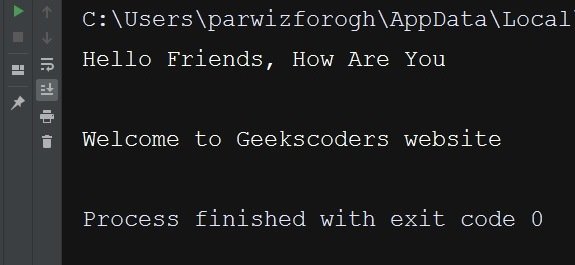
If you want to remove the blank spaces than you can use rstrip() function.
1 2 3 4 5 |
file_name = 'mfile.txt' with open(file_name) as file_object: for line in file_object: print(line.rstrip()) |