In this Python Tutorial we are going to learn about Python For & While Loop, so we will learn how you can create for and while loops in Python, first we want to learn about for loop.
Python For Loop
Python For Loop is used for iterating over a (a list, a tuple, a dictionary, or a string). or we can say in Python for loop is a control flow statement that allows you to iterate over a sequence of elements, such as a list, tuple, or string and perform set of operations on each element in the sequence.
The general syntax of a for loop in Python is:
1 2 |
for variable in sequence: # code to be executed for each element |
Here variable is new variable that takes on the value of each element in the sequence one by one and the indented block of code under for statement is executed for each iteration of the loop.
OK first we want to loop though a list, we are going to create a Python List and after that we want to iterate on the list.
1 2 3 4 5 |
#loop a list languages = ["Python", "C++", "Java", "C#", "Ruby"] for lan in languages: print(lan) |
This is the result.

Also you can do looping on a String.
1 2 3 4 5 |
#loop string name = "GeeksCoders" for i in name: print(i) |
Now let’s iterate over Python Dictionary and find key and value.
1 2 3 4 5 6 7 8 9 10 |
user = { 'username': 'Parwiz', 'first': 'Forogh', 'email': 'par@gmail.com', } for key, value in user.items(): print(f"Key: {key}") print(f"Value: {value}") |
This is the result.

Also you can loop through all dictionary items.
1 2 3 4 5 6 7 8 9 10 |
programing_lang = { 'Parwiz':'Python', "Bob":'Java', "John":'C#', "Habib":'C++' } for name, language in programing_lang.items(): print(f"{name} Favorite Language Is {language}") |
This is the result.
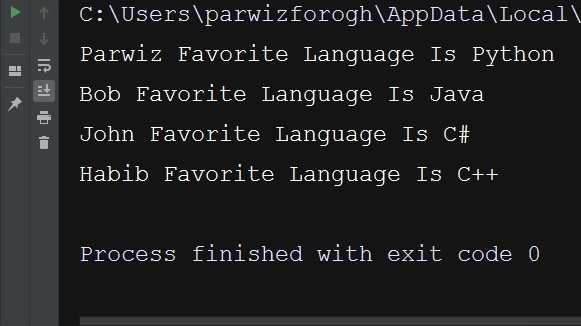
Now let’s loop through values in Dictionary.
1 2 3 4 5 6 7 8 9 10 |
programing_lang = { 'Parwiz':'Python', "Bob":'Java', "John":'C#', "Habib":'C++' } for language in programing_lang.values(): print(language) |
This is the result.
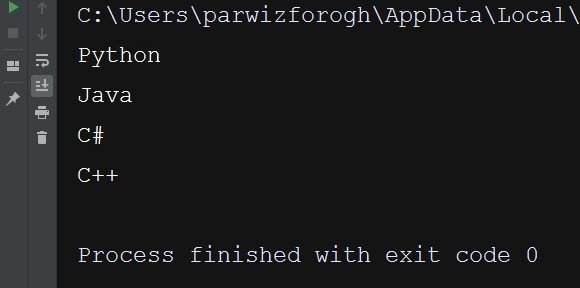
Let’s iterate over a tuple.
1 2 3 4 5 6 |
#iterating over a tuple fruits = ("Banana", "Pear", "Apple", "Melon") for fruit in fruits: print(fruit) |
This is the result.

You can use for loop to perform any kind of operation on sequence of elements such as searching for specific value, counting the number of elements that meet certain condition or modifying the elements in the sequence. by understanding how to use for loops effectively you can simplify your code and perform complex operations on data with easy.
Python While Loop
With the while loop we can execute a set of statements as long as a certain condition is true, or we can say that in Python a while loop is control flow statement that allows you to repeatedly execute a block of code while a certain condition is true. while loop continues to execute the block of code as long as the condition remains true.
The general syntax of while loop in Python is:
1 2 |
while condition: # code to be executed while the condition is true |
Here condition is a boolean expression that is evaluated before each iteration of the loop. If the condition is True, the block of code under the while statement is executed. After each iteration, the condition is checked again, and if it remains True the loop continues to execute.
This is a basic example
1 2 3 4 5 |
number = 1 while number < 8: print(number) number += 1 |
First we have created a number variable, and after that we are using while, now when the condition is true we are printing our number and incrementing the number, when the condition became false we don’t print the number.
This is the result.

This is another example.
1 2 3 4 5 6 7 |
prompt = "Enter a message, if you want to 'quite' press q : " message = "" while message != 'q': message = input(prompt) print(message) |
This is the result.
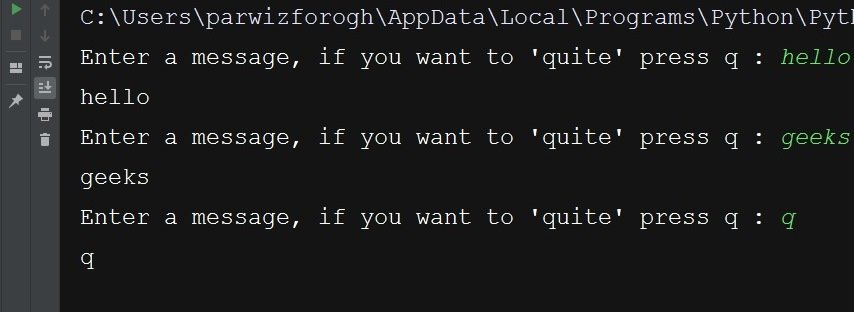
With the break statement we can stop the loop even if the while condition is true.
1 2 3 4 5 6 |
number = 1 while number < 7: print(number) if number == 4: break number += 1 |
With the continue statement we can stop the current iteration, and continue with the next.
1 2 3 4 5 6 |
number = 0 while number < 7: number += 1 if number == 3: continue print(number) |
You can use while loop to perform any kind of operation that requires repeated execution while certain condition is met such as searching for specific value or processing a stream of data. However you should be careful to ensure that the loop condition will eventually become False to avoid an infinite loop that will run forever.
Does Python have Do While Loop ?
Python does not have built in do while loop like some other programming languages. In do while loop, the loop body is executed at least once before the loop condition is checked.
However, in Python, you can achieve the same behavior using while loop with break statement to exit the loop. for example:
1 2 3 4 5 |
while True: # code to be executed at least once # ... if some_condition: break |
In this example the loop condition is always True, so the loop will execute code inside the loop body at least once. the loop body can contain any code that needs to be executed, and if statement with the break statement inside can be used to exit the loop when certain condition is met.
While Python does not have a built in do while loop but it provides flexible constructs like while loops and conditional statements that can be used to achieve the same behavior in a way that is compatible with Python’s syntax and design principles.
Difference between For and While Loops in Python
The main difference between for and while loops in Python is the way they iterate through sequence of elements.
for loop is used to iterate through sequence of elements, such as list, tuple or string and perform set of operations on each element in the sequence. the loop variable takes on the value of each element in the sequence one by one, and the loop body is executed for each element in the sequence.
while loop on the other hand is used to repeatedly execute block of code while certain condition is true. the loop body is executed repeatedly as long as the condition remains true. the condition is typically boolean expression that is evaluated before each iteration of the loop.
In general you should use for loop when you know the number of iterations in advance, such as when iterating through a sequence of known length. for loops are usually more concise and readable than while loops in this case.
You should use while loop when you don’t know the number of iterations or when you need to repeat block of code until certain condition is met. while loops can be more flexible and powerful than for loops in this case, but they can also be more complex and harder to read and debug.
This is an example that illustrates the difference between for and while loops in Python:
1 2 3 4 5 6 7 8 9 10 |
# Using for loop to print the elements of list numbers = [1, 2, 3, 4, 5] for n in numbers: print(n) # Using while loop to print the elements of list i = 0 while i < len(numbers): print(numbers[i]) i += 1 |
In this example both loops accomplish the same task of printing the elements of list. However for loop is more concise and easier to read, while the while loop is more verbose and requires an additional variable (i) to keep track of the index of the current element in the list.