in this Python Tutorial we want to learn about Python Function, so Python Function is block of code that performs specific task. when you want to do a particular task that you’ve defined in a function, you call the function responsible for it. If you need to perform that task multiple times throughout your program, you don’t need to type all the code for the same task again and again, you can just call the function dedicated to handling that task, and the call tells Python to run the code inside the function. You’ll find that using functions makes your programs easier to write and read.
Now let’s create a simple Python Function example in our Python Tutorial, you can use def keyword for creating functions in python.
1 2 3 4 5 |
def greeting(): print("Hello Friends, Welcome to GeeksCoders.com") greeting() |
In the above code we have created a simple function or method in python, after creating of the python function, if you want to use from this function you need to call that.
For example you are in a situation that you need this code again now you don’t need to write this code again and you don’t need define this function again, you can just call this function as much as you want.
1 2 3 4 5 6 7 8 |
def greeting(): print("Hello Friends, Welcome to GeeksCoders.com") greeting() greeting() greeting() greeting() |
Passing Information to Function
Sometimes you need to pass some information in the function, now for that we can call function parameters. so we can say that a piece of information that the function needs to do its job is called function parameters. in this example we have just created a simple function and we have passed username as parameter in the function, now when you call your function you need to give a username as parameter, if you don’t do this you will receive error.
1 2 3 4 5 |
def greet_user(username): print(f'Welcome : {username}') greet_user('Geekscoders') greet_user('Parwiz') |
Run the code and this is the result.
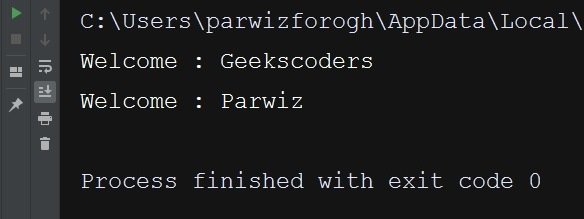
Also you can pass multiple parameters to a function, in this example we are going to pass two parameters in the function, now when you call your function you need to pass two parameters.
1 2 3 4 5 6 7 |
def print_name(firstname, lastname): full_name = firstname + lastname print(f'Your Full Name Is : {full_name}') print_name('Geeks', 'Coders') |
Default Parameters
Some times you need to add default parameters in Python functions, in this example we want to add two parameters in the function, and the second parameter has a default value, now when you call your function you can just pass one value to the function, and it will take the second parameter as default parameter.
1 2 3 4 5 6 7 |
def add(x, y = 5): sum = x + y print(sum) add(4) add(7,3) |
Returning a Value
A function doesn’t always have to display its output directly. Instead, it can process some data and then return a value or set of values. The value the function returns is called a return value. The return statement takes a value from inside a function and sends it back to the line that is called by the function. in this example we want to create a function that takes two parameters, after that we want to add these two parameters together and return that.
1 2 3 4 5 6 7 |
def print_full(firstname, lastname): full_name = firstname + lastname return full_name myname = print_full('Geeks', ' Coders ') print(f'Full Name Is : {myname}') |
Run the code and this is the result.
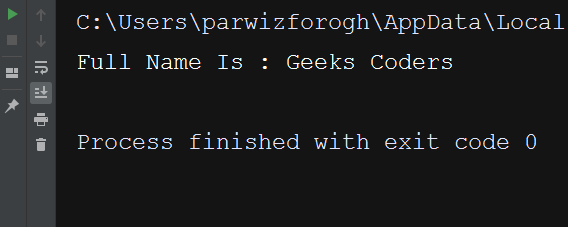
Returning a Dictionary in Function
A function can return any kind of value, we have learned that how you can return a simple string, but some times you need to return a dictionary, now let’s create the example.
1 2 3 4 5 6 7 8 9 10 11 |
def person_info(firstname, lastname): person = {'first':firstname, 'last':lastname} return person myname = person_info('Geeks', 'Coders') print(myname) print(myname['first']) print(myname['last']) |
Passing a List to Python Function
Sometimes you need to pass a list to the function, so in this example we are passing a list in to the python function.
1 2 3 4 5 6 7 8 9 |
def greet(names): for name in names: message = f'Welcome, {name}' print(message) usernames = ['Geekscoders', 'Admin', 'Forogh', 'John'] greet(usernames) |
Run the code and this is the result.

Passing Arbitrary Numbers of Arguments in Python Functions
Sometimes you won’t know ahead of time how many arguments a function needs to accept. fortunately, Python allows a function to collect an arbitrary number of arguments from the calling statement. For example, consider a function that print numbers. It needs to accept number , but you can’t know ahead of time how many numbers will be added for printing. the function in the following example has one parameter, *numbers, but this parameter collects as many arguments as the calling line provides. you can use asterisks for doing this.
1 2 3 4 |
def add(*numbers): print(numbers) add(1,2,3,4,5,6,7,8,9) |
The asterisk in the parameter name *numbers tells Python to make an empty tuple called numbers and pack whatever values it receives into this tuple. The print() call in the function body produces output showing that Python can handle a function call with one value and a call with different values. It treats the different calls similarly. Note that Python packs the arguments into a tuple, even if the function receives only one value.
Now you can use for loop and iterate on the tuple.
1 2 3 4 5 |
def add(*numbers): for number in numbers: print(number) add(1,2,3,4,5,6,7,8,9) |
Functions are an essential part of programming in Python and are used extensively to break down complex tasks into smaller, more manageable pieces of code.