About Lesson
In this React Native lesson we are going to learn about React Native useReducer Hooks, according to React Documentation it is an alternative to useState. accepts a reducer of type (state, action) => newState, and returns the current state paired with a dispatch method. (If you’re familiar with Redux, you already know how this works.).
This is my ReducerHooks.js.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
import React, {useReducer} from 'react' import {Button, View, Text} from 'react-native'; const initialState = {count:0} const reducer = (state, action) => { switch (action.type) { case "increment": return {count: state.count + 1}; case 'decrement': return {count: state.count - 1}; } } function ReducerHooks() { const [state, disptach] = useReducer(reducer, initialState) return ( <View style = {{padding:40}}> <View style = {{flexDirection:"row"}}> <Button title = "Increment" onPress = {() => disptach({type:'increment'})} /> <Button title = "Decrement" onPress = {() => disptach({type:'decrement'})} /> </View> <Text style = {{fontSize:25, padding:20}}>Count:{state.count}</Text> </View> ) } export default ReducerHooks |
This is our App.js file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
import React from 'react'; import ReducerHooks from './components/ReducerHooks'; function App() { return ( <ReducerHooks/> ); } export default App |
Run your application and this is the result.
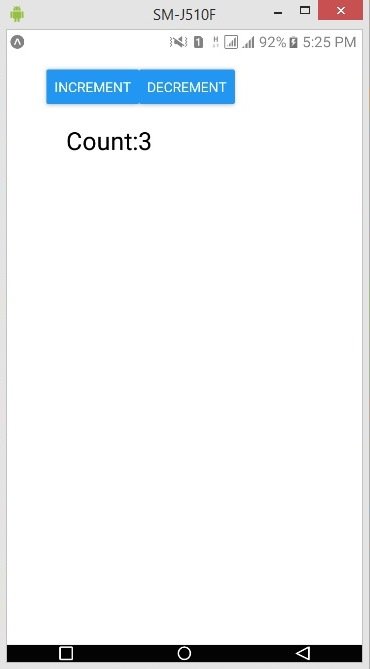