About Lesson
In this React Native lesson we are going to learn about React Native ViewPager, it is a simple component that allows the user to swipe between multiple pieces of content.
Installation
First of all we need to install viewpager component, by default there is a built in viewpager, but that is deprecated, we are going to install the another version.
1 |
npm install react-native-pager-view |
This is our ReactPager.js, in here we want to swipe between three images, make sure that you have already added some images in your working director.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
import React from 'react' import {StyleSheet, View, Text, Image} from 'react-native'; import PagerView from 'react-native-pager-view'; function ReactPager() { return ( <PagerView style={styles.pagerView} initialPage={0}> <View key="1"> <Image style = {{width:"100%", height:200, marginTop:50}} source = {require('../img/cake.jpg')}/> </View> <View key="2"> <Image style = {{width:"100%", height:200, marginTop:50}} source = {require('../img/cake2.jpg')}/> </View> <View key="3"> <Image style = {{width:"100%", height:200, marginTop:50}} source = {require('../img/cake3.jpg')}/> </View> </PagerView> ) } const styles = StyleSheet.create({ pagerView: { flex: 1, }, }); export default ReactPager |
This is our App.js.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import React from 'react'; import ReactPager from './components/ReactPager'; function App() { return ( <ReactPager/> ); } export default App |
Run your application and this is the result.
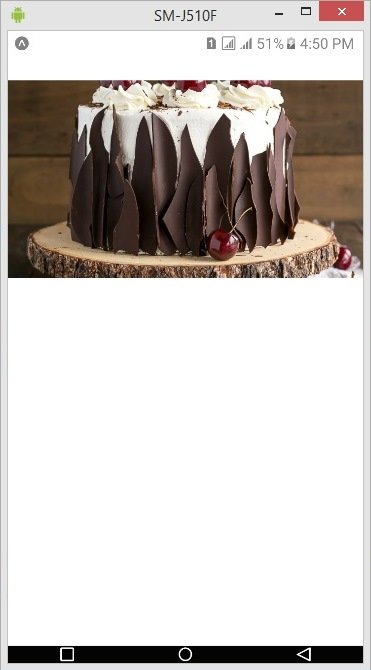