About Lesson
in this TKinter lesson we want to learn how to Add Items to TKinter TabWidget, in the previous lesson we have learned that how you can create TabWidget, and that was an empty tabwidget, now we want to add some item widgets to the tabwidget.
This is the complete code for this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
from tkinter import * from tkinter import ttk class Window(Tk): def __init__(self): super(Window, self).__init__() self.title("Tab Widget In TKinter") self.minsize(500,400) self.wm_iconbitmap("myicon.ico") tab_control = ttk.Notebook(self) self.tab1 = ttk.Frame(tab_control) tab_control.add(self.tab1, text = "Example One") self.tab2 = ttk.Frame(tab_control) tab_control.add(self.tab2, text = "Example Two") tab_control.pack(expan =1, fill = "both") self.add_widgets() def add_widgets(self): #tab one label_frame = LabelFrame(self.tab1, text = "Example One Tab") label_frame.grid(column = 0, row = 0, padx = 8, pady = 4) label = Label(label_frame, text = "Enter Your Name : ") label.grid(column = 0, row = 0) text_edit = Entry(label_frame, width = 25) text_edit.grid(column = 1, row = 0) label2 = Label(label_frame, text = "Enter Your Password : ") label2.grid(column = 0, row = 1) text_edit2 = Entry(label_frame, width = 25) text_edit2.grid(column = 1, row =1 ) #tabe two label_frame2 = LabelFrame(self.tab2, text = "Second Tab Example") label_frame2.grid(column = 0, row = 0) label = Label(label_frame2, text="Enter Your Name : ") label.grid(column=0, row=0) text_edit = Entry(label_frame2, width=25) text_edit.grid(column=1, row=0) label2 = Label(label_frame2, text="Enter Your Password : ") label2.grid(column=0, row=1) text_edit2 = Entry(label_frame2, width=25) text_edit2.grid(column=1, row=1) window = Window() window.mainloop() |
In here we are going to create our tab control and tabwidget.
1 2 3 4 5 6 7 8 |
tab_control = ttk.Notebook(self) self.tab1 = ttk.Frame(tab_control) tab_control.add(self.tab1, text = "Example One") self.tab2 = ttk.Frame(tab_control) tab_control.add(self.tab2, text = "Example Two") tab_control.pack(expan =1, fill = "both") |
Using this code you can add items, basically we are going to create some labels with text entry.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#tab one label_frame = LabelFrame(self.tab1, text = "Example One Tab") label_frame.grid(column = 0, row = 0, padx = 8, pady = 4) label = Label(label_frame, text = "Enter Your Name : ") label.grid(column = 0, row = 0) text_edit = Entry(label_frame, width = 25) text_edit.grid(column = 1, row = 0) label2 = Label(label_frame, text = "Enter Your Password : ") label2.grid(column = 0, row = 1) text_edit2 = Entry(label_frame, width = 25) text_edit2.grid(column = 1, row =1 ) |
Run the complete code and this is the result
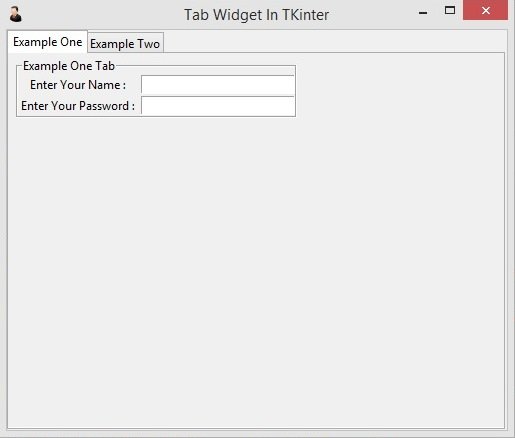