In this lesson we are going to learn about TKinter Insert Data to MySQL, we want to lean how you can insert some data to the database, in the previous lesson we have learned that how you can connect your tkinter application to mysql database.
We have already created our database at name of tkdb, now we want to create a table with three fields id, name and password.
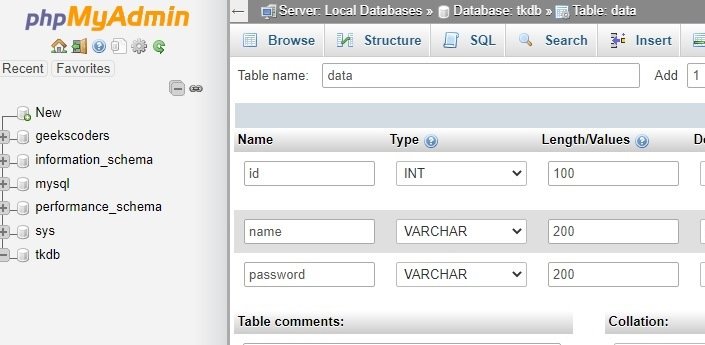
This is the complete code for this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
from tkinter import * from tkinter import ttk import MySQLdb as mdb from tkinter import messagebox as msg class Window(Tk): def __init__(self): super(Window, self).__init__() self.title("Mysql Database Connection") self.minsize(500,400) self.wm_iconbitmap("myicon.ico") self.label_frame = ttk.LabelFrame(self, text = "Inserting Data") self.label_frame.grid(column = 0, row =0, padx = 20, pady = 20) self.create_widgets() def create_widgets(self): label = Label(self.label_frame, text="Enter Your Name : ") label.grid(column=0, row=0) self.text_edit = Entry(self.label_frame, width=25) self.text_edit.grid(column=1, row=0) label2 = Label(self.label_frame, text="Enter Your Password : ") label2.grid(column=0, row=1) self.text_edit2 = Entry(self.label_frame, width=25) self.text_edit2.grid(column=1, row=1) button = ttk.Button(self.label_frame, text = "Insert Data", command = self.db_connect) button.grid(column = 1, row = 2) def db_connect(self): con = mdb.connect("localhost", "root", "", "tkdb") cur = con.cursor() cur.execute("INSERT INTO data(name, password)" "VALUES('%s', '%s')" % (''.join(self.text_edit.get()), ''.join(self.text_edit2.get()))) msg.showinfo("Inserting Data", "Data Inserted Successfully") window = Window() window.mainloop() |
basically we have create two labels with two text input field.
1 2 3 4 5 6 7 8 9 10 11 |
label = Label(self.label_frame, text="Enter Your Name : ") label.grid(column=0, row=0) self.text_edit = Entry(self.label_frame, width=25) self.text_edit.grid(column=1, row=0) label2 = Label(self.label_frame, text="Enter Your Password : ") label2.grid(column=0, row=1) self.text_edit2 = Entry(self.label_frame, width=25) self.text_edit2.grid(column=1, row=1) |
This is the button that we want to insert the data.
1 2 3 |
button = ttk.Button(self.label_frame, text = "Insert Data", command = self.db_connect) button.grid(column = 1, row = 2) |
First we need to connect our application to the MySQL database, and after that we need to create the cursor object.
1 2 |
con = mdb.connect("localhost", "root", "", "tkdb") cur = con.cursor() |
Now we need to execute our query.
1 2 3 |
cur.execute("INSERT INTO data(name, password)" "VALUES('%s', '%s')" % (''.join(self.text_edit.get()), ''.join(self.text_edit2.get()))) |
After inserting the data we want to show a messagebox.
1 |
msg.showinfo("Inserting Data", "Data Inserted Successfully") |
Run the complete code insert the data this will be the result.

Also if you check your wamp server, we have the inserted data.
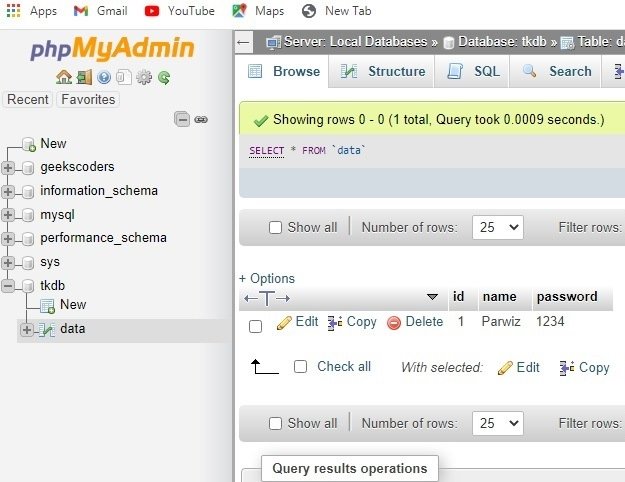