About Lesson
In this TKinter Tutorial we are going to learn about TKinter FileDialog, we want to learn how you can browse a file in tkinter using FileDialog widget.
This is the complete code for this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
from tkinter import * from tkinter import ttk from tkinter import filedialog class Window(Tk): def __init__(self): super(Window, self).__init__() self.title("Dialog Widget") self.minsize(500,400) self.wm_iconbitmap("myicon.ico") self.label_frame = ttk.LabelFrame(self, text = "Open A File") self.label_frame.grid(column = 0, row = 1, padx = 20, pady = 20) self.create_button() def create_button(self): button = ttk.Button(self.label_frame, text = "Browse A File", command = self.fileDialog) button.grid(column = 1, row = 1) def fileDialog(self): file_name = filedialog.askopenfilename(initialdir = "/", title = "Select A File", filetype = (("jpeg", "*.jpg"), ("All Files", "*.*"))) label = ttk.Label(self.label_frame, text = "") label.grid(column = 1, row = 2) label.configure(text = file_name) window = Window() window.mainloop() |
In here we have created a label frame, also we have added the label frame in a grid layout.
1 2 |
self.label_frame = ttk.LabelFrame(self, text = "Open A File") self.label_frame.grid(column = 0, row = 1, padx = 20, pady = 20) |
This is the button for browsing the file.
1 2 3 |
button = ttk.Button(self.label_frame, text = "Browse A File", command = self.fileDialog) button.grid(column = 1, row = 1) |
We can use askopenfilename() for browsing in tkinter, and you need to give some parameters like initial directory, title and file type.
1 2 3 |
filedialog.askopenfilename(initialdir = "/", title = "Select A File", filetype = (("jpeg", "*.jpg"), ("All Files", "*.*"))) |
In this label we want to show the result name of our browsing file.
1 2 3 |
label = ttk.Label(self.label_frame, text = "") label.grid(column = 1, row = 2) label.configure(text = file_name) |
Run the complete code and this is the result.
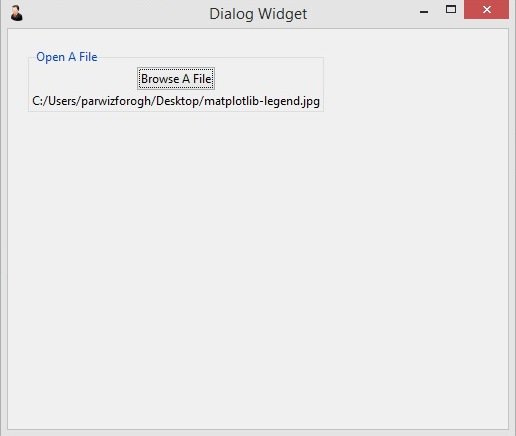