About Lesson
In this TKinter Tutorial we are going to learn create TKinter LabelFrame, basically labelframe is a containter, it is used for complex window layout.
This is the complete code for this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
import tkinter as tk from tkinter import ttk class Window(tk.Tk): def __init__(self): super(Window, self).__init__() self.title("LabelFrame") self.minsize(350,350) self.wm_iconbitmap("myicon.ico") self.labelFrame = ttk.LabelFrame(self, text = "TKinter Label Frame") self.labelFrame.grid(column = 0, row = 7, padx = 20, pady = 40) self.create_labels() def create_labels(self): ttk.Label(self.labelFrame, text = "Python").grid(column = 0, row = 0) ttk.Label(self.labelFrame, text="Java").grid(column=0, row=1) ttk.Label(self.labelFrame, text="C++").grid(column=0, row=2) window = Window() window.mainloop() |
This code is used for creating of the LabelFrame in TKinter, also you need to give the title for the labelframe.
1 |
self.labelFrame = ttk.LabelFrame(self, text = "TKinter Label Frame") |
These are the widgets that we want to add in the labelframe, basically we want to add three labels in the labelframe.
1 2 3 |
ttk.Label(self.labelFrame, text = "Python").grid(column = 0, row = 0) ttk.Label(self.labelFrame, text="Java").grid(column=0, row=1) ttk.Label(self.labelFrame, text="C++").grid(column=0, row=2) |
Run the complete code and this is the result.
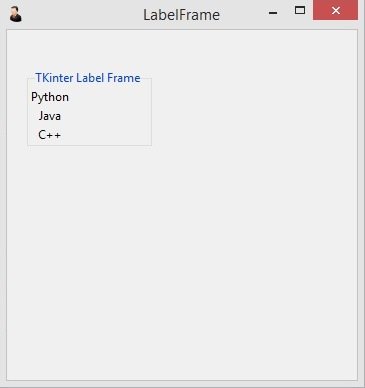