In this Pygame article we want to learn about Pygame Display: Setting Up Game Window, Pygame is popular library for creating games and multimedia applications using Python. the first thing in Pygame is the window itself. in this article we want to cover how to use Pygame module to create and set up the game window, so let’s talk about Pygame
What is Pygame ?
Pygame is cross platform set of Python modules designed for writing video games. it provides functionalities for creating 2D games with graphics, sound and user input. Pygame was designed to make it easier for people to make games using Python and it provides simple and easy to learn API for game development. Pygame is built on top of the SDL (Simple DirectMedia Layer) library, which provides low level access to audio, keyboard, mouse, joystick and graphics hardware. it means that Pygame games can be run on multiple platforms, including Windows, macOS, Linux and Raspberry Pi, without requiring any modification to the code. Pygame provides different features for game development, including support for image and sound loading and playback, sprite animation, input handling and game loop management. it also provides set of simple but powerful abstractions for game components, such as sprites and collision detection which make it easier to build games quickly.
Pygame Display: Setting Up Game Window
First of all we need to install Pygame
1 |
pip install pygame |
For setting up game window, we need to use display module. this module provides functions and classes for managing the game window. this is an example code to create and set up the game window:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import pygame pygame.init() screen_width = 640 screen_height = 480 screen = pygame.display.set_mode((screen_width, screen_height)) pygame.display.set_caption("Geekscoders.vcom - Pygame Window") # Game loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Update game logic # Draw game objects pygame.display.update() pygame.quit() |
In the above code we have imported Pygame module and initialize it using pygame.init(). after that we have defined width and height of the game window, and creates game window using pygame.display.set_mode(). we also set the caption of the game window using pygame.display.set_caption(). this sets the title of the game window.
Next part of the code is game loop. this loop runs until the running variable is set to False. inside the loop we handle events using pygame.event.get(). we checks if the pygame.QUIT event is triggered, which happens when the user clicks on the close button of the game window. if this event occurs, we set the running variable to False, which exits the game loop.
And lastly we update the game logic and draw game objects. pygame.display.update() function updates the game window with any changes made to the game objects.
Run the code and this will be the result
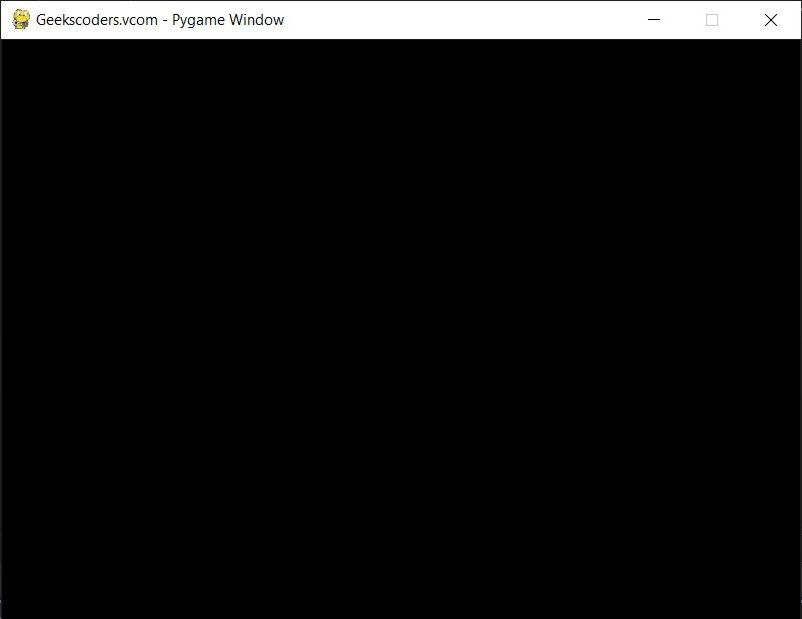