In this Python GUI Programming article we are going to learn about Create Desktop Applications with Python, Python is powerful programming language that has become increasingly popular over years. one of the reasons for this popularity is it is ability to create graphical user interfaces (GUIs) for desktop applications. in this article we are going to learn how Python can be used for GUI programming and how to create desktop applications with Python, when it comes to Python, you have different options for build GUI Applications, we are going to learn about two libraries TKinter and PyQt6.
GUI Programming with Python
Python has different libraries that can be used for GUI programming including Tkinter, PyQt, PySide and wxPython. Tkinter is the most commonly used library for creating GUIs in Python. it is included in the standard Python distribution and is easy to learn and it makes it an excellent choice for beginners, also we have PyQt6, it is third party package.
Creating Desktop Applications with Python
Desktop applications are programs that run on user computer and typically have graphical user interface. Python can be used to create desktop applications that can run on Windows, macOS and also Linux. In this article we are going to explore how to create simple desktop application using Tkinter and PyQt6 that is third party library.
Now let’s first create our Python Desktop Application with TKinter
Import Tkinter : the first step in creating desktop application with Tkinter is to import library. To do this we use following code:
1 |
import tkinter as tk |
this code imports Tkinter library and gives it alias “tk” to make it easier to use in our code.
Create Window : the next step is to create window for our application. we can do this using following code:
1 2 3 |
root = tk.Tk() root.title("My Application") root.geometry("300x200") |
this code creates new window object using Tk() method and sets its title to My Application. we also set size of the window to 300 pixels wide and 200 pixels tall using geometry() method.
Add Widgets : Once we have created our window, we can add widgets to it, such as buttons, labels and text boxes. this is an example of how to add button to our window:
1 2 |
button = tk.Button(root, text="Click Me!") button.pack() |
This code creates new button object using Button() method and sets its text to Click Me. after that we add button to our window using the pack() method.
Run Application: final step is to run our application. we can do this using the following code:
1 |
root.mainloop() |
This code runs main loop of our application, which listens for events such as button clicks and updates GUI accordingly.
This is our complete code for Python GUI with TKinter
1 2 3 4 5 6 7 |
import tkinter as tk root = tk.Tk() root.title("My Application") root.geometry("300x200") button = tk.Button(root, text="Click Me!") button.pack() root.mainloop() |
Run the code and this will be the result
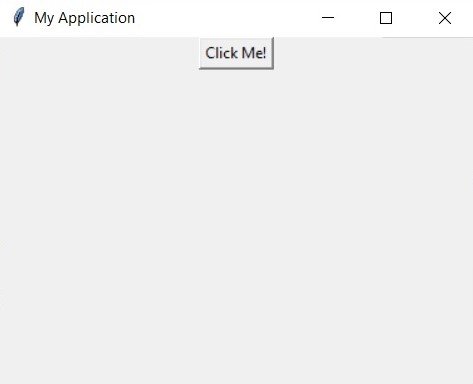
We have learned that how we can build simple desktop application with Python and TKinter, so Tkinter is most commonly used library for GUI programming in Python and can be used to create different desktop applications.
These are the steps for creating Desktop Applications with Python
Install PyQt6 : before we can use PyQt6 we need to install it. this can be done using pip and you can use this command for installation of PyQt6.
1 |
pip install PyQt6 |
Import PyQt6: after installing PyQt6, we can import it into our code using the following code:
1 |
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton |
by using this code imports we can import QApplication, QWidget and QPushButton classes from PyQt6.QtWidgets module.
Create Window : next step is to create window for our application. we can do this using following code:
1 2 3 4 |
app = QApplication([]) window = QWidget() window.setWindowTitle("My Application") window.setGeometry(100, 100, 300, 200) |
This code creates new QApplication object and new QWidget object. after that we set title of the window to My Application and it is size to 300 pixels wide and 200 pixels tall using setGeometry() method.
Add Widgets : after creating our basic window now we can add widgets to it, such as buttons, labels and text boxes. this is an example of how to add button to our window:
1 2 |
button = QPushButton("Click Me!", window) button.move(100, 80) |
This code creates new QPushButton object with the text Click Me and adds it to our window using window argument. after that we set position of the button to 100 pixels from the left and 80 pixels from the top using move() method.
ShowWindow : final step is to show our window. we can do this using following code:
1 2 |
window.show() app.exec() |
This code shows our window using show() method and starts the main event loop using exec() method.
This is complete code for Python GUI Programming with PyQt6
1 2 3 4 5 6 7 8 9 10 |
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton app = QApplication([]) window = QWidget() window.setWindowTitle("My Application") window.setGeometry(100, 100, 300, 200) button = QPushButton("Click Me!", window) button.move(100, 80) window.show() app.exec() |
Run above code and this will be the result.
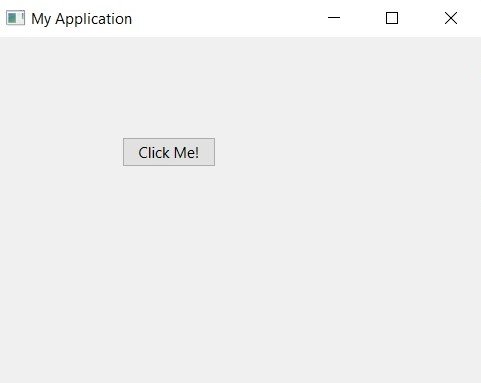
Learn More on TKinter
- PyQt5 QTableWidget Tutorial: Create a Dynamic Table
- Create GUI Applications with Python & TKinter
- Python TKinter Layout Management
- How to Create Label in TKinter
- How to Create Buttin in Python TKinter
- Build Music Player in Python TKinter
- Python GUI Programming with TKinter
- TKinter VS PyQt, Which one is Good
- Creating Custom Widgets in TKinter
In this article, we have learned how to create Python simple desktop application using PyQt6 and TKinter. PyQt6 is powerful and flexible library for GUI programming in Python and offers many features and tools for creating desktop applications. with PyQt6 creating desktop applications with Python has never been easier, also Tkinter is the most commonly used library for GUI programming in Python and can be used to create different types of desktop applications.