In this Java article we want to learn about Control Flow Statements in Java, Control flow statements are fundamental aspect of any programming language. they are used to control the flow of execution of program. in Java there are several types of control flow statements that you can use to control execution flow of your program. in this article we want to talk about these statements and how they are used.
Types of Control Flow Statements
There are three types of control flow statements in Java:
decision-making |
looping |
branching |
Decision-making statements are used to make decisions based on certain conditions. there are two types of decision-making statements in Java.
if statements |
switch statements |
Looping statements are used to execute a block of code repeatedly. there are three types of looping statements in Java:
for loops |
while loops |
do-while loops |
Branching statements are used to transfer control of a program to different part of the program. there are two types of branching statements in Java:
break statements |
continue statements |
If Statements
if statement is used to make a decision based on certain condition. if the condition is true, then the code in the if statement is executed. if the condition is false, then the code in the if statement is skipped.
1 2 3 4 5 6 7 8 9 10 11 12 |
public class HelloWorld { public static void main(String[] args) { int x = 5; if (x < 10) { System.out.println("x is less than 10"); } } } |
In this example condition is x < 10. since x is less than 10, the code within the if statement is executed, which prints out x is less than 10.
This is the result
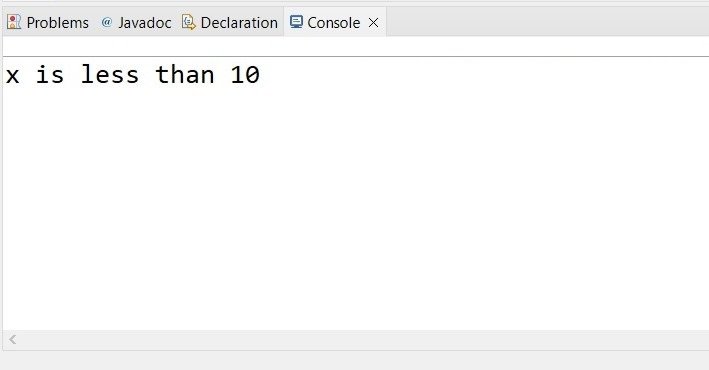
Switch Statements
switch statement is used to make a decision based on a certain value. switch statement evaluates the value of an expression and then executes the code associated with the matching case.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
public class HelloWorld { public static void main(String[] args) { int day = 4; String dayName; switch (day) { case 1: dayName = "Monday"; break; case 2: dayName = "Tuesday"; break; case 3: dayName = "Wednesday"; break; case 4: dayName = "Thursday"; break; case 5: dayName = "Friday"; break; case 6: dayName = "Saturday"; break; case 7: dayName = "Sunday"; break; default: dayName = "Invalid day"; break; } System.out.println(dayName); } } |
In this example the value of day is 4. the code within the case labeled 4 is executed, which sets dayName to Thursday. break statement is used to exit the switch statement once a case has been matched.
For Loops
for loop is used to execute a block of code repeatedly for fixed number of times. the syntax of for loop is like this:
1 2 3 |
for (initialization; condition; update) { // code to be executed } |
The initialization statement is executed once before the loop starts. condition is checked before each iteration of the loop. if the condition is true, then the code within the loop is executed. also update statement is executed after each iteration of the loop.
This is an example of for loop.
1 2 3 |
for (int i = 0; i < 10; i++) { System.out.println(i); } |
In this example the loop will execute 10 times. i variable is initialized to 0, and the loop will continue to execute as long as i is less than 10.
This will be the result
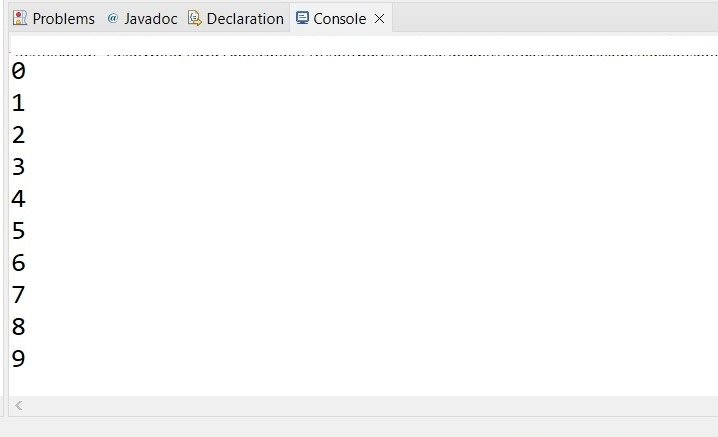
While Loops
while loop is used to execute a block of code repeatedly as long as certain condition is true. syntax of a while loop is like this, the code within the loop will continue to execute as long as the condition is true.
1 2 3 |
while (condition) { // code to be executed } |
This is the example of while loop in java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public class HelloWorld { public static void main(String[] args) { int i = 0; do { System.out.println(i); i++; } while (i < 10); } } |
In this example the loop will execute 10 times. the code within the loop will continue to execute as long as i is less than 10. i++ statement is used to increment the value of i after each iteration of the loop.
Break Statements
break statement is used to exit a loop or switch statement before the loop or switch statement has completed its full execution. syntax of a break statement is like this:
1 |
break; |
This is the complete code within for loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public class HelloWorld { public static void main(String[] args) { for (int i = 0; i < 10; i++) { if (i == 5) { break; } System.out.println(i); } } } |
In this example the loop will execute 5 times. when i is equal to 5, break statement is executed which exits the loop before it has completed its full execution.
This will be the result
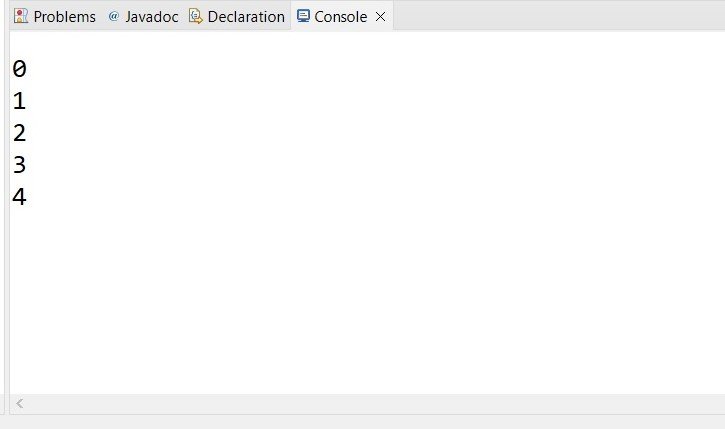
Continue Statements
continue statement is used to skip over certain iteration of a loop. syntax of a continue statement is like this:
1 |
continue; |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public class HelloWorld { public static void main(String[] args) { for (int i = 0; i < 10; i++) { if (i == 5) { continue; } System.out.println(i); } } } |
In this example the loop will execute 10 times, but the number 5 will be skipped over. when i is equal to 5, continue statement is executed, which skips over the code within the loop for that iteration.
So we can say that In Java control flow statements are used to control the flow of execution of a program. there are several types of control flow statements, including decision-making statements, looping statements and branching statements.