In this Django Tutorial we are going to talk about Django Include Tag, Include tag loads a template and renders it with the current context. this is a way of “including” other templates within a template. the template name can either be a variable or a hard-coded (quoted) string, in either single or double quotes. for example in our application we want a navigation bar, now you can create a new template and include that template in your base.html.
We want to create a navbar.html in our templates folder, and we are going to bring the bootstrap nav bar code from our base.html in to navbar.html.
mysite/templates/navbar.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<nav class="navbar navbar-expand-lg navbar navbar-dark bg-dark"> <a class="navbar-brand" href="#">GeeksCoders</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavAltMarkup" aria-controls="navbarNavAltMarkup" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarNavAltMarkup"> <div class="navbar-nav"> <a class="nav-link active" href="#">Home <span class="sr-only">(current)</span></a> <a class="nav-link" href="#">Polls</a> </div> </div> </nav> |
Now you can use django include tag for including your navbar.html, we want to include this file in our base.html. because we want the navbar for all the pages that are extending from our base html file.
1 |
{% include 'navbar.html' %} |
So this is our base.html file and we have added the include tag in our body tag.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<!DOCTYPE html> {% load static %} <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %}{% endblock %}</title> <!-- CSS --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/css/bootstrap.min.css" > <link rel="stylesheet" href="{% static 'css/style.css' %}"> </head> <body> {% include 'navbar.html' %} {% block body %} {% endblock %} <!-- jQuery and JS bundle w/ Popper.js --> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" > </script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/js/ bootstrap.bundle.min.js" ></script> </body> </html> |
Now these are our rest of files for the project.
mysite/templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Welcome to GeeksCoders.com</h1> <p>Tutorial Number 10 - Django Include Tag</p> </div> {% endblock %} |
mysite/polls/views.py
1 2 3 4 5 6 7 8 |
from django.shortcuts import render # Create your views here. def Index(request): context = {} return render(request, 'index.html', context) |
mysite/polls/models.py
1 2 3 4 5 6 7 8 9 10 11 |
from django.db import models # Create your models here. class Question(models.Model): text = models.CharField(max_length=100) pub_date = models.DateTimeField('date published') def __str__(self): return self.text |
mysite/polls/admin.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
from django.contrib import admin from .models import Question # Register your models here. #first way #admin.site.register(Question) #second way @admin.register(Question) class QuestionModel(admin.ModelAdmin): list_filter = ('text', 'pub_date') list_display = ('text', 'pub_date') date_hierarchy = ('pub_date') ordering = ('pub_date', 'text') |
mysite/static/css/style.css
1 2 3 4 5 6 7 8 9 10 11 |
h1 { color:green } p { color:red } |
mysite/urls.py
1 2 3 4 5 6 7 |
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('', include('polls.urls')) ] |
mysite/polls/urls.py
1 2 3 4 5 |
from django.urls import path from .views import Index urlpatterns = [ path('', Index, name = 'index') ] |
Run your django project and go to http://localhost:8000/, and this will be the result, you can see that how easily we have included our navbar.
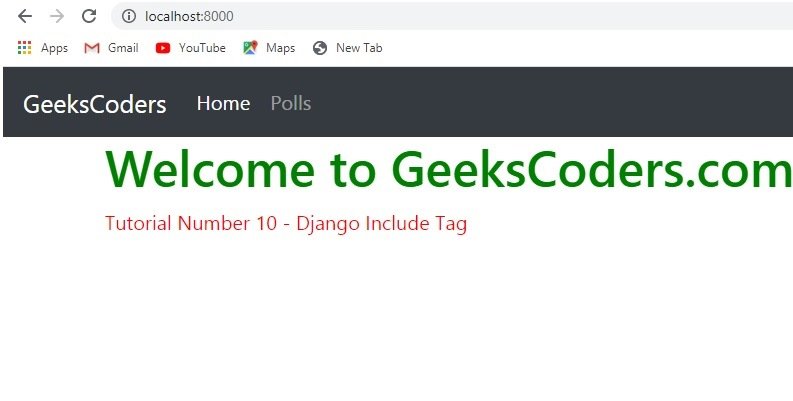