In this article i want to show you Rendering Database Data in Django Templates, so as you know we are using SQLite database and we have inserted some data in to our database, now we want to render that data in to our html template, also we will learn that how you can use for loop for iterating over the data.
Now open your views.py file, if you remember we have a Polls view function, so we want to retrieve our data in this view function and after that we want to render this data in to our polls_list.html.
1 2 3 4 5 6 |
def Polls(request): polls = Question.objects.all() context = {'polls':polls} return render(request, 'polls_list.html', context) |
In the above view function first we have retrieved our data from Question model, we are using all() method because i want to get all the data and after that i want to send the data through context variable to our html file.
So now this is our complete views.py file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
from django.shortcuts import render from .models import Question # Create your views here. def Index(request): context = {} return render(request, 'index.html', context) def Polls(request): polls = Question.objects.all() context = {'polls':polls} return render(request, 'polls_list.html', context) |
Now open your polls_list.html file, because we want to retrieve the data in this file, so add this code. in here we are using for loop to iterate over the data in our database and render that data in to a list.
1 2 3 4 5 6 7 |
{% for poll in polls %} <ul class="list-group"> <li class="list-group-item"> {{poll}} </li> </ul> {% endfor %} |
This is our complete code for polls_list.html.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
{% extends 'base.html' %} {% block title %} Polls List {% endblock %} {% block body %} <div class="container"> <h1>Polls List Page</h1> {% for poll in polls %} <ul class="list-group"> <li class="list-group-item"> {{poll}} </li> </ul> {% endfor %} </div> {% endblock %} |
Now run the code and this is the result.
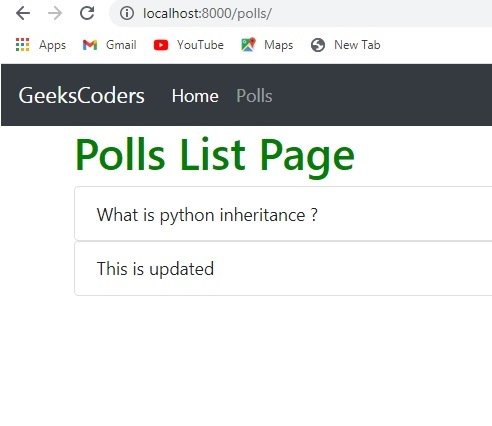
Rest of the file for the tutorial.
mysite/templates/base.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<!DOCTYPE html> {% load static %} <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %}{% endblock %}</title> <!-- CSS --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/css/bootstrap.min.css" > <link rel="stylesheet" href="{% static 'css/style.css' %}"> </head> <body> {% include 'navbar.html' %} {% block body %} {% endblock %} <!-- jQuery and JS bundle w/ Popper.js --> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" > </script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/js/ bootstrap.bundle.min.js" ></script> </body> </html> |
mysite/templates/navbar.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<nav class="navbar navbar-expand-lg navbar navbar-dark bg-dark"> <a class="navbar-brand" href="{% url 'index' %}">GeeksCoders</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavAltMarkup" aria-controls="navbarNavAltMarkup" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarNavAltMarkup"> <div class="navbar-nav"> <a class="nav-link active" href="{% url 'index' %}">Home <span class="sr-only">(current)</span></a> <a class="nav-link" href="{% url 'polls_list' %}">Polls</a> </div> </div> </nav> |
mysite/templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Welcome to GeeksCoders.com</h1> <p>Tutorial Number 12 - Render Database Data</p> </div> {% endblock %} |
mysite/polls/models.py
1 2 3 4 5 6 7 8 9 10 11 |
from django.db import models # Create your models here. class Question(models.Model): text = models.CharField(max_length=100) pub_date = models.DateTimeField('date published') def __str__(self): return self.text |
mysite/polls/admin.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
from django.contrib import admin from .models import Question # Register your models here. #first way #admin.site.register(Question) #second way @admin.register(Question) class QuestionModel(admin.ModelAdmin): list_filter = ('text', 'pub_date') list_display = ('text', 'pub_date') date_hierarchy = ('pub_date') ordering = ('pub_date', 'text') |
mysite/polls/urls.py
1 2 3 4 5 6 |
from django.urls import path from .views import Index, Polls urlpatterns = [ path('', Index, name = 'index'), path('polls/', Polls, name = 'polls_list') ] |
mysite/urls.py
1 2 3 4 5 6 7 |
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('', include('polls.urls')) ] |