In this lesson we want to learn about Python PySide6 QPrintDialog, PySide6 provides QPrintDialog class that simplifies the process of printing documents. in this lesson we are going to cover the basics of using QPrintDialog in PySide6 application.
Introduction to QPrintDialog
QPrintDialog is dialog box that allows user to select printer, configure printing options and initiate printing of document. when the user closes the dialog, QPrintDialog provides the selected printer and printing options to your application.
This is simple example that demonstrates how to use QPrintDialog in PySide6:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
from PySide6.QtWidgets import QApplication, QMainWindow, QTextEdit import sys from PySide6.QtGui import QAction from PySide6.QtPrintSupport import QPrintDialog class Example(QMainWindow): def __init__(self): super().__init__() # create QTextEdit widget to display the document self.textEdit = QTextEdit(self) self.setCentralWidget(self.textEdit) # create "Print" action and connect it to the print function printAction = QAction('Print', self) printAction.triggered.connect(self.print) # add the "Print" action to the file menu fileMenu = self.menuBar().addMenu('File') fileMenu.addAction(printAction) # set the window title and size self.setWindowTitle('Print Dialog Example') self.setGeometry(300, 300, 350, 300) def print(self): # create a QPrintDialog instance and execute it printDialog = QPrintDialog() if printDialog.exec() == QPrintDialog.Accepted: # if the user accepts, print the document self.textEdit.print(printDialog.printer()) if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() ex.show() sys.exit(app.exec()) |
In this example we have create new QTextEdit widget and set it as the central widget of our main window. we also create Print action that is added to the File menu and connect it to custom print() function.
When user clicks the Print action, print() creates new QPrintDialog instance and displays it to the user. if the user accepts the dialog, the QTextEdit.print() method is called with the selected printer, which initiates the printing of the document.
Run the complete code and this will be the result.
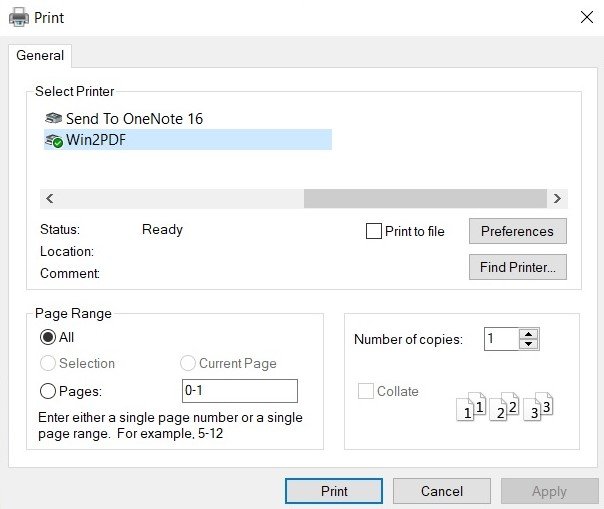
Final Thoughts
QPrintDialog class provides an easy way to allow users to print documents from your PySide6 application. with its simple API and built-in support for selecting printers and printing options, QPrintDialog can greatly simplify the process of printing for both you and your users.