In this Python Tutorial we are going to learn about Python Variables, Python Variables are fundamental concept in Python programming. they are used to store data in memory that can be accessed and manipulated throughout program.
1 2 3 4 5 6 7 8 9 |
c = 25 x = 2.5 name = "Parwiz" a = 'A' print(c) print(c) print(name) print(a) |
So in here we have created four different variables with different data types, for example we have integer variable, we have floating variable, we have string and also character variables, and you can see that we have not defined the types for these variables.
And this is the result.
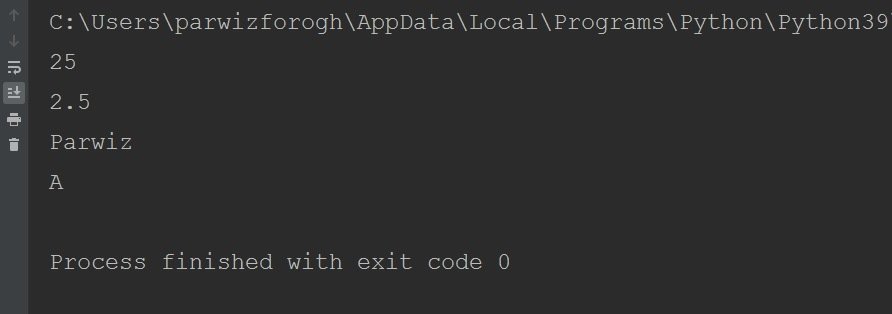
To define new Variables in Python, we simply assign a value to a label. For example, this is how we create a variable called count, which contains an integer value of 10.
1 2 3 |
count = 10 print(count) print(" Count Value is ", count) |
We can define several variables in one line, but this is usually considered bad style:
1 |
count, result, total = 2,2,2 |
Naming Rules for Python Variables
when you are going to create variables in python, you need to obey some rules for the name of the variable.
- Variable names can contain only letters, numbers, and underscores.
They can start with a letter or an underscore, but not with a number.
For example, you can call a variable count_1 but not 1_count. - You can not add spaces in the variable names, it is not allowed to add
spaces in variable names. but you can use underscore in the variables names. - You can not use Python keywords and function names as variable names;
do not use words that is reserved in Python for example print.
What is Python Variable scope ?
Python variable scope refers to the area of a program where a variable can be accessed or used. it determines where in the code the variable is visible and where it can be modified.
There are two types of variable scope in Python: local and global.
- Local variable scope: Variables declared inside a function have local scope, which means they are only accessible within that function. when the function ends, the local variables are destroyed and their values are no longer accessible.
- Global variable scope: Variables declared outside of any function have global scope, which means they can be accessed and modified throughout the entire program. However, it’s important to note that variables declared inside a function can also have global scope if they are declared as global variables using the “global” keyword.
It’s generally considered good practice to minimize the use of global variables as they can make it difficult to understand and maintain the code. Instead, it’s recommended to use local variables as much as possible, and pass data between functions using arguments and return values.
This is an example of how variable scope works in Python:
1 2 3 4 5 6 7 8 9 10 |
x = 10 # global variable def my_func(): y = 5 # local variable print(x) # can access global variable print(y) # can access local variable my_func() print(x) # can access global variable print(y) # NameError: name 'y' is not defined |
In this example variable x has global scope and can be accessed both inside and outside function my_func. the variable y has local scope and can only be accessed within function my_func. when function ends the variable y is destroyed and its value is no longer accessible. when we try to access y outside of function we get NameError because it’s undefined in that scope.
What is Python Variable re assignment
Python variable re-assignment refers to process of changing the value of variable after it has been initialized. this allows you to update value stored in variable based on the needs of your program.
In Python you can re assign variable by simply assigning new value to it using the assignment operator “=”.
This is an example
1 2 3 4 5 |
x = 10 # assign value 10 to variable x print(x) # prints 10 x = 20 # re-assign value 20 to variable x print(x) # prints 20 |
In this example we have first assigned the value 10 to variable x. after that we have printed value of x which is 10. and then re assign the value 20 to x using the “=” operator and print the new value of x which is 20.
It’s important to note that re assigning variable can change its data type. for example:
1 2 3 4 5 |
x = 10 # assign integer valu 10 to variable x print(x) # prints 10 x = "hello" # re assign the string "hello" to variable x print(x) # prints "hello" |
In this example we start by assigning the integer value 10 to x. after that re assign string hello to x which changes the data type of the variable from integer to string.
It’s important to use variable re assignment carefully to avoid accidentally overwriting the value of a variable or changing its data type in a way that causes errors in your program.
What is Best practices working with Python Variables ?
These are some best practices for working with Python variables:
- Use descriptive variable names: Choose variable names that clearly describe data they represent. this makes code easier to read and understand.
- Avoid using single letter variable names: Unless the variable is being used in very localized context, it is best to avoid using single letter variable names like x or y. Instead use descriptive variable names that convey meaning.
- Declare variables at the point of first use: Declare variables as close to the point where they are first used as possible. this makes it easier to understand where variables are being used and reduces the chance of accidentally re using the same variable name for different purposes.
- Use the correct data type for the task: Use appropriate data type for the data you are working. for example use integers for whole numbers and floats for numbers with decimal points.
- Use constants for values that don’t change: If value is never intended to be changed during the execution of the program, consider using constant to represent it. Constants are typically written in all caps with underscores separating words (e.g. MY_CONSTANT).
- Initialize variables before use: Always initialize variables with value before using them in your code. this helps prevent errors that can occur if you try to use variable that has not been assigned value.
- Minimize the use of global variables: Whenever possible try to use local variables rather than global variables. this makes it easier to understand and maintain the code.
- Use variable re assignment carefully: While variable re assignment can be useful, overuse of this feature can make the code difficult to read and understand. instead try to use separate variables for different tasks.
By following these best practices, you can write Python code that is easier to read, understand, and maintain, so hopefully you enjoyed from Python Tutorial – Python Variables lesson.