In this article we want to talk about Python PyOpenGL: A Guide to High-Performance 3D Graphics in Python.
Introduction
PyOpenGL is Python binding to the OpenGL graphics API. it provides powerful and flexible platform for creating 3D games and applications. with PyOpenGL you can leverage the power of OpenGL to create powerful 3D graphics, animations and visual effects. in this article we are going to explore the key features of PyOpenGL and how you can use them to create high-performance 3D graphics in Python.
What is PyOpenGL?
PyOpenGL is cross platform library that provides low level API for creating 3D graphics. it is built on top of the OpenGL API and provides Python interface to OpenGL’s capabilities. PyOpenGL is designed to be highly efficient and fast and it is an ideal choice for creating high performance 3D graphics.
Key Features of PyOpenGL
- Cross-platform compatibility: PyOpenGL is compatible with multiple operating systems including Windows, macOS and Linux. and it is an ideal choice for creating cross platform 3D graphics applications.
- Powerful API: PyOpenGL provides powerful and flexible API for creating 3D graphics. with PyOpenGL, you can create 3D models, textures and animations with easy.
- High performance: PyOpenGL is designed to be highly efficient and fast. it provides low level API for creating 3D graphics, which allows for maximum performance and efficiency.
- Large community: PyOpenGL has large and active community of users and developers. This makes it easy to find help and resources when you need it.
How to Install PyOpenGL ?
For installing PyOpenGL, you need to have Python and pip installed on your computer. after you have these prerequisites, you can install PyOpenGL by running the following command in your terminal:
1 |
pip install PyOpenGL |
Basic Example of PyOpengl
PyOpenGL provides low level API for creating 3D graphics. for using PyOpenGL, you need to have basic understanding of the OpenGL API and 3D graphics programming. this is basic example of how to create a 3D cube using PyOpenGL:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
import pygame from pygame.locals import * from OpenGL.GL import * from OpenGL.GLU import * vertices = ( (1, -1, -1), (1, 1, -1), (-1, 1, -1), (-1, -1, -1), (1, -1, 1), (1, 1, 1), (-1, -1, 1), (-1, 1, 1) ) edges = ( (0,1), (0,3), (0,4), (2,1), (2,3), (2,7), (6,3), (6,4), (6,7), (5,1), (5,4), (5,7) ) def Cube(): glBegin(GL_LINES) for edge in edges: for vertex in edge: glVertex3fv(vertices[vertex]) glEnd() def main(): pygame.init() display = (800,600) pygame.display.set_mode(display, DOUBLEBUF|OPENGL) gluPerspective(45, (display[0]/display[1]), 0.1, 50.0) glTranslatef(0.0,0.0, -5) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit() glRotatef(1, 3, 1, 1) glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT) Cube() pygame.display.flip() pygame.time.wait(10) main() |
This code should render 3D cube that can be rotated by pressing the quit button.
This is a step-by-step explanation of the code:
1 2 3 4 |
import pygame from pygame.locals import * from OpenGL.GL import * from OpenGL.GLU import * |
These lines imports the necessary libraries and modules. Pygame is library for creating games in Python, while OpenGL is cross platform API for rendering 2D and 3D graphics. GL and GLU are sub-libraries in the OpenGL library.
1 2 3 4 5 6 7 8 9 10 |
vertices = ( (1, -1, -1), (1, 1, -1), (-1, 1, -1), (-1, -1, -1), (1, -1, 1), (1, 1, 1), (-1, -1, 1), (-1, 1, 1) ) |
This line defines 8 vertices of 3D cube. Each tuple represents vertex and its x, y, and z coordinates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
edges = ( (0,1), (0,3), (0,4), (2,1), (2,3), (2,7), (6,3), (6,4), (6,7), (5,1), (5,4), (5,7) ) |
This line defines the edges of the cube, represented by pairs of vertices. each tuple is an edge, with its two endpoints represented by indices into the vertices array.
1 2 3 4 5 6 |
def Cube(): glBegin(GL_LINES) for edge in edges: for vertex in edge: glVertex3fv(vertices[vertex]) glEnd() |
This line defines the edges of the cube, represented by pairs of vertices. each tuple is an edge, with its two endpoints represented by indices into the vertices array.
1 2 3 4 5 6 |
def Cube(): glBegin(GL_LINES) for edge in edges: for vertex in edge: glVertex3fv(vertices[vertex]) glEnd() |
function Cube is used to render the cube on the screen. glBegin and glEnd calls define the start and end of sequence of vertices that define set of geometric primitives (in this case, lines). glVertex3fv call is used to specify vertex in 3D space and its position. for loops iterate through each edge and vertex in the edges array, and glVertex3fv call is used to specify the position of each vertex in 3D space.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
def main(): pygame.init() display = (800,600) pygame.display.set_mode(display, DOUBLEBUF|OPENGL) gluPerspective(45, (display[0]/display[1]), 0.1, 50.0) glTranslatef(0.0,0.0, -5) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit() glRotatef(1, 3, 1, 1) glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT) Cube() pygame.display.flip() pygame.time.wait(10) main() |
This is the main function of the program. It initializes Pygame, sets up the display window and do the rotation job.
Run the complete code and this will be the result.

Now let’s draw triangle with Pyopengl.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
import pygame from pygame.locals import * from OpenGL.GL import * from OpenGL.GLU import * vertices = ( (1, -1, 0), (-1, -1, 0), (0, 1, 0) ) def Triangle(): glBegin(GL_TRIANGLES) for vertex in vertices: glVertex3fv(vertex) glEnd() def main(): pygame.init() display = (800,600) pygame.display.set_mode(display, DOUBLEBUF|OPENGL) gluPerspective(45, (display[0]/display[1]), 0.1, 50.0) glTranslatef(0.0,0.0, -5) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit() glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT) Triangle() pygame.display.flip() pygame.time.wait(10) main() |
This program creates single triangle using the vertices defined in the vertices
array. Triangle
function uses glBegin
and glEnd
to specify the start and end of sequence of vertices that define set of geometric primitives (in this case, triangles). glVertex3fv
call is used to specify a vertex in 3D space and its position.
Run the complete code and this will be the result
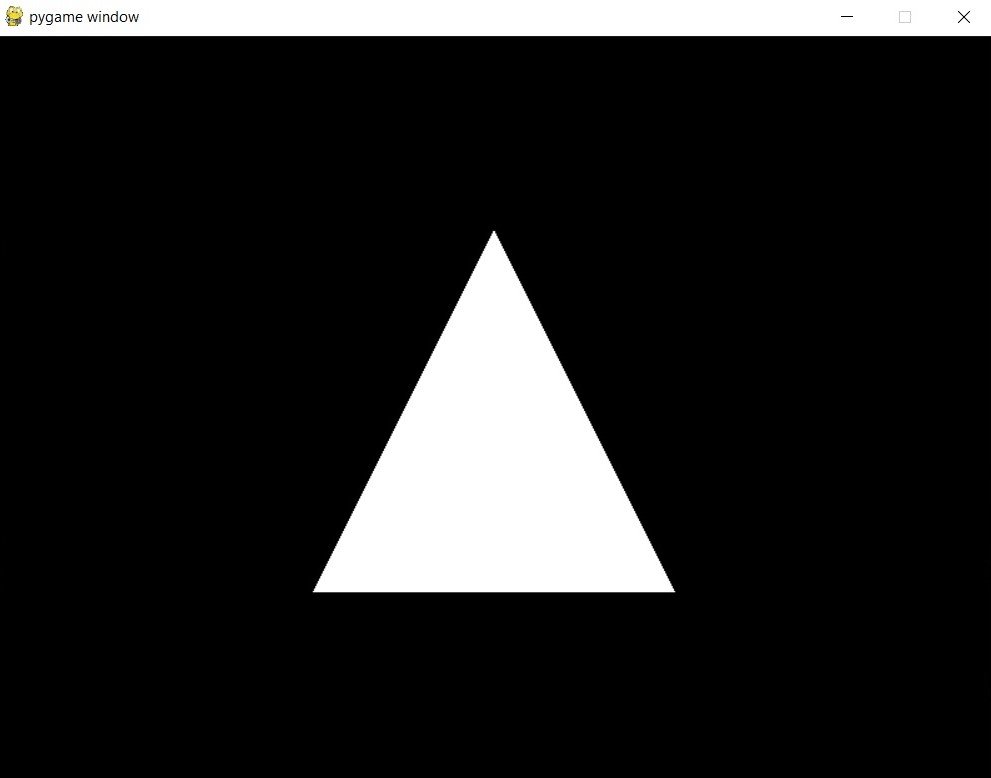
Now let’s talk about modern opengl and we want to create our triangle using modern opengl
What is Modern OpenGL ?
Modern OpenGL, also known as OpenGL 3.x and higher is version of the OpenGL graphics API that provides significant improvements and additional features over previous versions. Modern OpenGL is designed to be more efficient, scalable and programmable than previous versions. it provides number of new capabilities such as improved shading, lighting and texturing as well as advanced features like programmable shaders and advanced buffer objects. the core idea behind modern OpenGL is to provide more flexible and programmable pipeline for graphics development, which allows for more advanced graphics and visualization capabilities.
Here is an example of how to draw triangle with modern OpenGL and PyOpenGL:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
import pygame import numpy as np from pygame.locals import * from OpenGL.GL import * vertex_shader_source = """ #version 330 in vec3 position; void main() { gl_Position = vec4(position, 1.0f); } """ fragment_shader_source = """ #version 330 out vec4 outColor; void main() { outColor = vec4(1.0f, 0.5f, 0.2f, 1.0f); } """ def create_shader(shader_type, source): shader = glCreateShader(shader_type) glShaderSource(shader, source) glCompileShader(shader) return shader def create_program(vertex_shader, fragment_shader): program = glCreateProgram() glAttachShader(program, vertex_shader) glAttachShader(program, fragment_shader) glLinkProgram(program) return program def main(): pygame.init() display = (800, 600) pygame.display.set_mode(display, DOUBLEBUF|OPENGL) vertex_shader = create_shader(GL_VERTEX_SHADER, vertex_shader_source) fragment_shader = create_shader(GL_FRAGMENT_SHADER, fragment_shader_source) program = create_program(vertex_shader, fragment_shader) vertices = np.array([-0.5, -0.5, 0.0, 0.5, -0.5, 0.0, 0.0, 0.5, 0.0], dtype=np.float32) VBO = glGenBuffers(1) glBindBuffer(GL_ARRAY_BUFFER, VBO) glBufferData(GL_ARRAY_BUFFER, vertices.nbytes, vertices, GL_STATIC_DRAW) position_location = glGetAttribLocation(program, "position") while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit() glClear(GL_COLOR_BUFFER_BIT) glUseProgram(program) glBindBuffer(GL_ARRAY_BUFFER, VBO) glEnableVertexAttribArray(position_location) glVertexAttribPointer(position_location, 3, GL_FLOAT, GL_FALSE, 0, None) glDrawArrays(GL_TRIANGLES, 0, 3) pygame.display.flip() pygame.time.wait(10) main() |
This program uses modern OpenGL and shaders to draw single triangle. the vertex shader is responsible for transforming the vertex data into screen space, and the fragment shader is responsible for coloring the fragments generated by the rasterization of the triangle. The `create_shader
Learn More on Python
- PyQt6: The Ultimate GUI Toolkit for Python
- Python: The Most Versatile Programming Language of the 21st Century
- Tkinter: A Beginner’s Guide to Building GUI Applications in Python
- PySide6: The Cross-Platform GUI Framework for Python
- The Ultimate Guide to Kivy: Building Cross-Platform Apps with Python
- Discover the Power of Django: The Best Web Framework for Your Next Project
- How to Earn Money with Python
- Why Flask is the Ideal Micro-Web Framework
- Python Pillow: The Ultimate Guide to Image Processing with Python
- Get Started with Pygame: A Beginner’s Guide to Game Development with Python