In this article we are going to talk about Getting Started with GUI Development in Python & PyQt6, so first of all let’s talk about GUI Development, Graphical User Interfaces (GUIs) are key component of modern software development. using GUI users can interact with software in easy and efficient way. Python is powerful programming language and it is used for different types of applications including GUI development. one of the most popular frameworks for building GUIs in Python is PyQt6. in this article we are going talk how to get started with GUI development in Python and PyQt6.
What is PyQt6 ?
PyQt6 is Python bindings for Qt application framework and runs on all different platforms supported by Qt including Windows, macOS, Linux, iOS and Android. so now let’s talk about Qt, Qt is cross platform application development framework widely used for building GUIs for desktop, mobile and embedded devices in C++ Programming Langauge. PyQt6 provides access to all the functionalities of Qt and this makes it powerful tool for building GUI applications in Python.
First of all we need to install PyQt6, and you can use pip for the installation.
1 |
pip install PyQt6 |
Now let’s create our simple GUI application with Python & PyQt6.
OK first of all we need to import our required modules and class from PyQt6
1 2 |
import sys from PyQt6.QtWidgets import QApplication, QWidget, QLabel, QPushButton |
After that we are goin got create a new class that inherits from the QWidget class. this will be our main window:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class MyApp(QWidget): def __init__(self): super().__init__() self.setWindowTitle('My PyQt6 Application') self.setGeometry(100, 100, 400, 300) self.label = QLabel('Hello World!', self) self.label.move(150, 50) self.button = QPushButton('Click me!', self) self.button.move(150, 100) self.button.clicked.connect(self.on_button_click) def on_button_click(self): self.label.setText('Button clicked!') |
In the above code we set the window title and size using setWindowTitle() and setGeometry() methods. also we have created label and button using the QLabel and QPushButton classes. after that we set the label text using the setText() method and connect the button click event to the on_button_click() method.
And finally we need to create an instance of our MyApp class and show the window:
1 2 3 4 5 |
if __name__ == '__main__': app = QApplication(sys.argv) ex = MyApp() ex.show() sys.exit(app.exec()) |
We have created an instance of QApplication class that us the main application object, also we have created an instance of our MyApp class. and call the show() method to display the window. and at the end we starts the event loop by calling app.exec() and exit the application when the loop terminates using sys.exit().
This is the complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
import sys from PyQt6.QtWidgets import QApplication, QWidget, QLabel, QPushButton class MyApp(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setWindowTitle('My PyQt6 Application') self.setGeometry(100, 100, 400, 300) self.label = QLabel('Hello World!', self) self.label.move(150, 50) self.button = QPushButton('Click me!', self) self.button.move(150, 100) self.button.clicked.connect(self.on_button_click) def on_button_click(self): self.label.setText('Button clicked!') if __name__ == '__main__': app = QApplication(sys.argv) ex = MyApp() ex.show() sys.exit(app.exec()) |
Run the code and this is the result
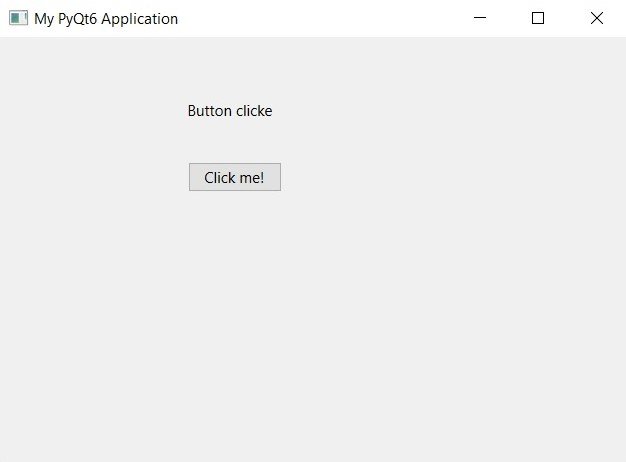
Learn More on Python GUI
- How to Create Label in PySide6
- How to Create Button in Python & PySide6
- How to Use Qt Designer with PySide6
- How to Add Icon to PySide6 Window
- How to Load UI in Python PySide6
- How to Create RadioButton in PySide6
- How to Create ComboBox in PySide6
- How to Create CheckBox in Python PySide6
- Responsive Applications with PyQt6 Multithreading
- Event Handling in Python and PyQt6
so PyQt6 provides powerful toolset for creating GUI applications in Python. with the help of this article you should have a basic understanding of how to get started with PyQt6 and create simple GUI application. however PyQt6 provides much more than what we have covered here.