In this lesson we want to learn about PyQt5: The Ultimate Guide to GUI Development with Python.
What is Python ?
Python is is one of the programming language and PyQt5 is one of the most popular libraries for GUI development. with PyQt5 you can build nice and user friendly applications for desktop and mobile devices. in this article we are going to take a closer look at PyQt5 and the benefits of using it for GUI development.
What is PyQt5 and How Does it Work ?
PyQt5 is set of Python bindings for the Qt libraries. it allows you to write GUI applications using Python and provides comprehensive set of widgets and tools to create nice user interfaces. PyQt5 uses the Qt Creator IDE to write, design and debug applications, and it makes it easy to develop complex applications with drag and drop interface.
Benefits of Using PyQt5 for GUI Development
There are several benefits to using PyQt5 for GUI development, including its cross platform compatibility, easy to use and large community of users. with PyQt5, you can develop applications that run on Windows, Linux and MacOS, ensuring that your applications are accessible to various audience. also PyQt5 is easy to learn, even for beginners, making it a great choice for those new to GUI development.
Getting Started with PyQt5
Getting started with PyQt5 is easy. all you need is Python installed on your computer and you can install PyQt5 using pip. after that you have PyQt5 installed, you can start writing your first PyQt5 application by following the tutorials available on the PyQt5 website.
Creating Stunning User Interfaces with PyQt5
With PyQt5, you can create nice user interfaces that provide seamless user experience. PyQt5 provides different widgets and tools, including buttons, checkboxes, comboboxes and many more, that you can use to build your user interface. also PyQt5 supports CSS, making it easy to style your user interface and add custom styles to your application.
How to install PyQt5 ?
Installing PyQt5 is simple process. this is how you can do it:
- Install Python: PyQt5 requires Python to be installed on your system. if you have not installed it, you can install latest version of Python from official Python website.
- Install pip: Pip is package manager for Python and it is used to install PyQt5. if pip is not installed you can check pip website for the installation instructions.
- Install PyQt5: After installing pip now you can install PyQt5 by running the following command in your terminal or command prompt:
1 |
pip install PyQt5 |
after completing the installation you can start using PyQt5 to build your GUI applications. you can also install additional packages to use with PyQt5, such as PyQt5 Designer which provides visual interface for designing your user interface.
Other Libraries for Python GUI Development
There are several other libraries available for GUI development in Python including:
- Tkinter: Tkinter is built in GUI toolkit for Python, and it provides simple and easy to use interface for developing GUI applications. Tkinter is good choice for beginners or for simple applications that don’t require more advanced features.
- wxPython: wxPython is powerful GUI library for Python and it provides rich set of widgets and tools for building complex applications. wxPython has large community of users and is well documented and it is great choice for more advanced applications.
- PyGTK: PyGTK is another GUI library for Python that provides large set of widgets and tools for building desktop applications. PyGTK is used by many popular and open source applications and it has large community of users and developers.
- Kivy: Kivy is an open source Python library for developing mobile and desktop applications. Kivy provides modern and flexible interface for GUI development and it supports both touchscreen and mouse based inputs.
These are just few of the many libraries available for GUI development in Python. and the best library for you will depend on your specific needs and requirements so it is good idea to try out few different libraries to see which one works best for you.
Difference Between PyQt5 and PyQt6
PyQt5 and PyQt6 are both sets of Python bindings for the Qt libraries, but there are some differences between them:
- Python Compatibility: PyQt5 is compatible with Python 2.x and 3.x, while PyQt6 is only compatible with Python 3.x.
- Qt Version: PyQt5 is based on Qt 5, while PyQt6 is based on Qt 6. Qt 6 includes several new features and improvements over Qt 5, such as improved performance, a more modern and flexible architecture, and support for high-resolution displays.
- API Changes: The API (Application Programming Interface) for PyQt6 has changed in some places compared to PyQt5, so code written for PyQt5 may need to be modified to work with PyQt6.
- Support: PyQt5 has been around for longer and has a larger user base, so there is more support available for it. PyQt6 is a newer library, so there may be less support available for it.
In general PyQt6 is newer and more modern version of PyQt, with improved performance and more flexible architecture, but it may not be fully backwards compatible with PyQt5 and there may be less support available for it. if you are starting new project, PyQt6 may be good choice, but if you have existing code written for PyQt5, you may want to stick with PyQt5 for now.
Learn More on Python
- PyQt6: The Ultimate GUI Toolkit for Python
- Python: The Most Versatile Programming Language of the 21st Century
- Tkinter: A Beginner’s Guide to Building GUI Applications in Python
- PySide6: The Cross-Platform GUI Framework for Python
- The Ultimate Guide to Kivy: Building Cross-Platform Apps with Python
- Discover the Power of Django: The Best Web Framework for Your Next Project
- How to Earn Money with Python
- Why Flask is the Ideal Micro-Web Framework
- Python Pillow: The Ultimate Guide to Image Processing with Python
- Get Started with Pygame: A Beginner’s Guide to Game Development with Python
- Python PyOpenGL: A Guide to High-Performance 3D Graphics in Python
- The Cross-Platform Game Development Library in Python
- Unleash the Power of Computer Vision with Python OpenCV
Example of PyQt5
This is an example of how to create basic window using PyQt5:
1 2 3 4 5 6 7 |
import sys from PyQt5.QtWidgets import QApplication, QWidget app = QApplication(sys.argv) window = QWidget() window.show() sys.exit(app.exec_()) |
This code creates simple window using PyQt5’s QApplication and QWidget classes. the QApplication class is the main class for creating PyQt5 application and the QWidget class is the base class for creating a window.
show() method is called on the window object to display the window on the screen. finally app.exec_() method is called to start the event loop for the application, which is necessary for handling user events (such as mouse clicks and keyboard presses).
This code creates basic and empty window that can be closed by clicking the close button in the top right corner of the window. to add more functionality and components to the window, you can use the many other classes provided by PyQt5, such as buttons, labels and layouts.
Run the complete code and this will be the result
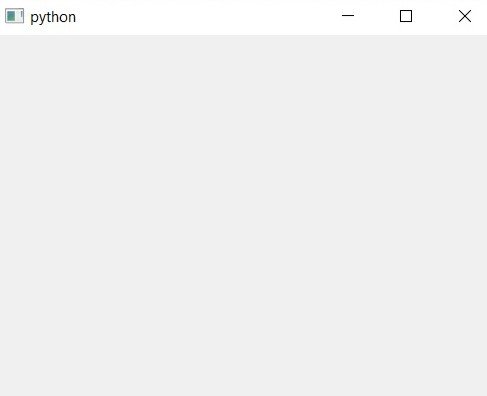
This is basic window in Python PyQt5, now let’s add QPushButton and QLabel in our GUI window.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import sys from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel app = QApplication(sys.argv) window = QWidget() button = QPushButton('Click Me!', window) label = QLabel('Hello, GeeksCoders!', window) button.move(100, 50) label.move(120, 100) window.show() sys.exit(app.exec_()) |
In this example the QPushButton and QLabel objects are created and added to the window. the move method is used to position the button and label within the window.
When the button is clicked the text in label will not change. to add functionality to the button, you can use PyQt5’s signal and slot mechanism, which allows you to connect the button’s clicked signal to a slot (a function that will be called when the button is clicked).
Run the complete code and this will be the result

In this code we don’t have layout, now let’s add some layouts to the window, Layout management in PyQt5 refers to process of arranging widgets within window in specific way. PyQt5 provides several classes for layout management, including QLayout, QBoxLayout, and QGridLayout, which allow you to arrange widgets in rows, columns and grids.
This is an example of how to add the QPushButton and QLabel from the previous answer to a layout:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import sys from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QVBoxLayout app = QApplication(sys.argv) window = QWidget() layout = QVBoxLayout() button = QPushButton('Click Me!') label = QLabel('Hello, GeeksCoders!') layout.addWidget(button) layout.addWidget(label) window.setLayout(layout) window.show() sys.exit(app.exec_()) |
In this example QVBoxLayout is created and added to the window using setLayout method. QVBoxLayout class arranges widgets in vertical column so the button and label will be displayed one above the other.
addWidget method is used to add QPushButton and QLabel widgets to the layout. widgets will be automatically resized and positioned within the window based on the layout’s rules.
Using layouts is an important part of PyQt5 programming, as it allows you to create flexible, resizable windows that will adapt to different screen sizes and resolutions.
Run the complete code and this will be the result.

Now let’s add signals and slots mechanism to our PyQt5 GUI Application, Signals and slots is a mechanism for communication between objects in PyQt5. It allows one object to send a signal (an event), which can be received by other objects known as slots. Slots can be any Python callable.
Signals and slots are used to connect the events triggered by user actions (such as button clicks or window resizing) with the appropriate response. For example, when a button is clicked, a clicked signal is emitted, and a connected slot is called to perform some action.
This is an example of how to use signals and slots in PyQt5:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
import sys from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QVBoxLayout app = QApplication(sys.argv) window = QWidget() button = QPushButton('Click Me!') label = QLabel('Hello, GeeksCoders!') button.clicked.connect(lambda: label.setText('Button was clicked!')) layout = QVBoxLayout() layout.addWidget(button) layout.addWidget(label) window.setLayout(layout) window.show() sys.exit(app.exec_()) |
In this example connect method is used to connect the clicked signal of the button to a lambda function that sets the text of the label to “Button was clicked!”. When the button is clicked, the signal is emitted and the connected slot is called, changing the text of the label.
Signals and slots provide a powerful and flexible way to add interactivity to your PyQt5 applications. They allow you to separate the code for handling events from the code for responding to those events, making your code cleaner and easier to maintain.
Run the complete code and click the button this will be the result.

Final Thoughts
PyQt5 is an excellent choice for GUI development, offering different benefits, including cross platform compatibility, easy to use and large community of users. whether you are new to GUI development or an experienced developer, PyQt5 provides the tools and resources you need to build stunning and user friendly applications. Start exploring PyQt5 today and discover the power of Python for GUI development.